People often view a DevOps roadmap as a set path to be followed to the letter.
The reality check, though, is when adherence to this “set path” fails to acknowledge individual challenges, leading to unforeseen obstacles.
From my experience with DevOps transformations, the most fruitful roadmaps are those that allow for flexibility and adaptability, constantly evolving in response to your team and project’s unique needs.
1. Learn Version Control (GIT)
Tools:
- Git
Resources:
- GitHub
- GitLab
- Bitbucket
Skills:
- Branching
- Merging
- Pull Requests
- Conflict Resolution
Version control is the backbone of DevOps. It allows multiple people to work on the same codebase without stepping on each other’s toes. Git is the most widely used version control system. Start by learning the basics: branching, merging, and resolving conflicts. Utilize platforms like GitHub and GitLab for practical experience.
# Example Git commands
git init
git add .
git commit -m "Initial commit"
git branch new-feature
git checkout new-feature
2. Learn a Programming Language
Languages:
- Python
- Go
- Ruby
- JavaScript
Skills:
- Scripting
- Automation
- Writing Efficient Code
Learning a programming language is crucial for scripting and automation tasks. Python is highly recommended due to its readability and vast library support. However, the choice of language can depend on your project needs and team preferences.
# Simple Python script
print("Hello, DevOps World!")
3. Learn Linux and Scripting
Skills:
- Command-Line Proficiency
- Shell Scripting (Bash)
- System Administration
Resources:
- Linux Distributions (Ubuntu, CentOS)
Mastering Linux and shell scripting is essential since many DevOps tools run on Linux. Get comfortable with the command line and practice writing shell scripts to automate routine tasks.
#!/bin/bash
echo "Hello, DevOps World!"
4. Learn Networking and Security
Skills:
- TCP/IP
- DNS
- HTTP/HTTPS
- Firewalls
- VPNs
- SSL/TLS
Resources:
- Networking Courses
- Security Best Practices
A solid understanding of networking and security principles is critical. Familiarize yourself with protocols like TCP/IP and HTTP/HTTPS, and learn how to secure your applications and infrastructure.
5. Learn Server Management and Configuration
Tools:
- Ansible
- Puppet
- Chef
Skills:
- Infrastructure Automation
- Configuration Management
Configuration management tools like Ansible, Puppet, and Chef help automate server setup and management. These tools allow you to manage large-scale deployments efficiently.
# Example Ansible playbook
- hosts: servers
tasks:
- name: Ensure Apache is installed
apt:
name: apache2
state: present
6. Learn Containers
Tools:
- Docker
Skills:
- Containerization
- Creating Docker Images
- Managing Containers
Containers are lightweight, portable, and consistent across various environments, making them perfect for DevOps. Docker is the go-to tool for containerization.
# Example Dockerfile
FROM python:3.8-slim-buster
COPY . /app
WORKDIR /app
RUN pip install -r requirements.txt
CMD ["python", "app.py"]
7. Learn Container Orchestration
Tools:
- Kubernetes
- Docker Swarm
Skills:
- Deploying
- Scaling
- Managing Containerized Applications
Once comfortable with containers, the next step is orchestration. Kubernetes is the most popular tool for managing containerized applications, ensuring they run smoothly across different environments.
8. Learn Infrastructure as Code (IaC)
Tools:
- Terraform
- CloudFormation
Skills:
- Writing and Managing Infrastructure as Code
- Automating Infrastructure Deployment
Infrastructure as Code (IaC) is a method to provision and manage computing infrastructure using code. Terraform and CloudFormation are powerful tools for this purpose.
# Example Terraform configuration
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
9. Learn Continuous Integration/Continuous Deployment (CI/CD)
Tools:
- Jenkins
- GitLab CI
- CircleCI
- Travis CI
Skills:
- Setting Up CI/CD Pipelines
- Automating Build, Test, and Deployment Processes
CI/CD automates the process of integrating and deploying code changes. Jenkins is a popular choice, but tools like GitLab CI and CircleCI are also excellent options.
// Example Jenkins pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building...'
}
}
stage('Test') {
steps {
echo 'Testing...'
}
}
stage('Deploy') {
steps {
echo 'Deploying...'
}
}
}
}
10. Learn Monitoring and Observability
Tools:
- Prometheus
- Grafana
- ELK Stack (Elasticsearch, Logstash, Kibana)
- Nagios
Skills:
- Monitoring System Performance
- Logging
- Alerting
- Visualization
Monitoring and observability tools help you understand your system’s health and performance. Prometheus and Grafana are excellent for monitoring and visualization.
11. Learn Cloud Providers
Providers:
- AWS
- Azure
- Google Cloud Platform (GCP)
Skills:
- Cloud Services
- Deployment
- Management
Cloud platforms like AWS, Azure, and GCP offer scalable and flexible resources. Learning to deploy and manage applications on these platforms is crucial for modern DevOps.
Cloud Services
Understanding cloud services is the foundation. Each provider offers a range of services for computing, storage, databases, and more.
- AWS:
- EC2: Virtual servers.
- S3: Scalable storage.
- RDS: Managed databases.
- Example: Set up an EC2 instance to host a web application and use S3 for storing media files.
- Azure:
- Virtual Machines: Similar to EC2.
- Blob Storage: Similar to S3.
- SQL Database: Managed databases.
- Example: Deploy a web app on Azure Virtual Machines and use Blob Storage for backups.
- GCP:
- Compute Engine: Virtual machines.
- Cloud Storage: Object storage.
- Cloud SQL: Managed databases.
- Example: Host a backend service on Compute Engine and store logs in Cloud Storage.
Deployment
Deploying applications in the cloud involves setting up the environment, configuring services, and automating the deployment process.
- AWS:
- Elastic Beanstalk: Simplifies deployment.
- CloudFormation: Infrastructure as Code.
- Example: Use Elastic Beanstalk to deploy a Node.js application, managing all underlying resources automatically.
- Azure:
- App Service: PaaS for web apps.
- Azure Resource Manager: Infrastructure as Code.
- Example: Deploy a Python web app using Azure App Service, allowing easy scaling and management.
- GCP:
- App Engine: PaaS for web apps.
- Deployment Manager: Infrastructure as Code.
- Example: Deploy a Java application using App Engine, benefitting from auto-scaling and integrated monitoring.
Management
Efficient cloud management includes monitoring, scaling, and optimizing resource usage.
- AWS:
- CloudWatch: Monitoring.
- Auto Scaling: Dynamic scaling.
- Example: Set up CloudWatch to monitor application performance and use Auto Scaling to adjust the number of EC2 instances based on traffic.
- Azure:
- Azure Monitor: Monitoring and diagnostics.
- Scale Sets: Dynamic scaling.
- Example: Use Azure Monitor to track metrics and logs, and Scale Sets to automatically adjust VM instances.
- GCP:
- Stackdriver: Monitoring and logging.
- Auto Scaling: Dynamic scaling.
- Example: Implement Stackdriver to monitor application health and configure auto-scaling for Compute Engine instances.
Practical Example
Suppose your team is working on a new microservices-based application. Here’s how you might approach using cloud providers:
- AWS:
- Deploy each microservice as a separate Docker container using ECS (Elastic Container Service).
- Store configuration data in S3 and use RDS for relational data.
- Azure:
- Use Kubernetes Service (AKS) for container orchestration.
- Store application logs in Blob Storage and use Azure SQL Database for persistent data.
- GCP:
- Deploy the microservices on GKE (Google Kubernetes Engine).
- Use Cloud Storage for file storage and Cloud SQL for database needs.
Mastering cloud providers like AWS, Azure, and GCP, you ensure your team can leverage the full potential of cloud services, streamline deployments, and efficiently manage resources.
12. Learn Software Engineering Practices
Skills:
- Agile Methodologies
- DevOps Culture
- Collaboration Between Development and Operations Teams
Adopting Agile methodologies and fostering a DevOps Culture are crucial for promoting collaboration and efficiency. This cultural shift is as important as technical skills. Let’s break down each element with practical examples.
Agile Methodologies
Agile methodologies, such as Scrum or Kanban, emphasize iterative progress, flexibility, and collaboration. Here’s how to implement them:
- Daily Stand-Ups:
- Hold short, daily meetings to discuss progress and obstacles.
- Keep everyone on the same page and address issues promptly.
- Sprint Planning:
- Plan work in short, manageable sprints (e.g., two weeks).
- Focus on delivering small, incremental changes.
- Retrospectives:
- After each sprint, review what worked and what didn’t.
- Make adjustments for the next sprint based on feedback.
DevOps Culture
Fostering a DevOps culture means breaking down silos between development and operations. Promote a mindset where everyone works together towards common goals.
- Shared Responsibilities:
- Developers take part in deployment processes.
- Operations team members get involved in the development cycle.
- Blameless Post-Mortems:
- When things go wrong, focus on learning and improvement, not blame.
- Encourage openness and trust within the team.
- Continuous Learning:
- Invest in ongoing training and development.
- Encourage team members to share new knowledge and skills.
Collaboration Between Development and Operations Teams
Effective collaboration is key to DevOps success. Here’s how to enhance it:
- Integrated Tools:
- Use shared tools (e.g., JIRA, Confluence) to facilitate communication and tracking.
- Ensure both teams have access to the same information.
- Joint Planning Sessions:
- Involve both development and operations teams in planning sessions.
- Align goals and understand each other’s challenges.
- Cross-Functional Teams:
- Create teams with members from both development and operations.
- Foster a sense of shared purpose and accountability.
Practical Example
Your team has just adopted Agile and DevOps practices. Here’s how you can see them in action:
- Daily Stand-Ups:
- Developers and operations staff meet every morning.
- They discuss current tasks, roadblocks, and next steps.
- Sprint Planning:
- Plan a two-week sprint to implement a new feature.
- Include tasks for both development (coding) and operations (deployment setup).
- Retrospectives:
- At the end of the sprint, the team reviews what went well and what needs improvement.
- Adjust the next sprint based on these insights.
- Blameless Post-Mortems:
- An outage occurs during deployment.
- The team conducts a post-mortem to understand the cause and prevent future issues without blaming individuals.
By embedding Agile methodologies, fostering a DevOps culture, and enhancing collaboration, you ensure that your team works efficiently and cohesively. This cultural shift, combined with technical prowess, leads to successful DevOps transformations.
Being adaptability and Flexibility
While the roadmap provides a structured approach, the real key to success in DevOps is flexibility and adaptability. Each team and project has unique challenges. Sticking rigidly to a set path can lead to obstacles and frustrations. Use the roadmap as a guideline, but be ready to pivot and adjust based on your specific needs.
Example Scenario
Imagine your team excels in Python but struggles with networking concepts. Pushing ahead with container orchestration might seem like the next step, but it’s more beneficial to first solidify your networking and security knowledge. Here’s a detailed approach:
- Identify the Gap:
- Acknowledge that networking is a weak area for your team.
- Adjust the Focus:
- Pause container orchestration efforts.
- Allocate time and resources to enhance networking skills.
- Practical Learning:
- Use real-world scenarios to practice setting up and securing networks.
- Implement firewalls, VPNs, and SSL/TLS in small projects.
- Integration:
- Gradually integrate these networking skills into your existing projects.
- Ensure everyone understands how these concepts apply to your DevOps practices.
- Review and Iterate:
- Regularly review your progress.
- Be ready to adapt your learning process as new challenges arise.
By focusing on your team’s specific needs, you build a strong, well-rounded foundation. This tailored approach ensures that your DevOps journey is smooth and efficient, leading to better overall outcomes.
Final Thoughts
The journey to mastering DevOps is not linear. It’s a dynamic and evolving process that requires constant learning and adaptation. By following this flexible roadmap and being open to change, you can navigate the complexities of DevOps and lead your team to success.
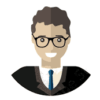
Noah is an accomplished technical author specializing in Operations and DevOps, driven by a passion ignited during his tenure at eBay in 2000. With over two decades of experience, Noah shares his transformative knowledge and insights with the community.
Residing in a charming London townhouse, he finds inspiration in the vibrant energy of the city. From his cozy writing den, overlooking bustling streets, Noah immerses himself in the evolving landscape of software development, operations, and technology. Noah’s impressive professional journey includes key roles at IBM and Microsoft, enriching his understanding of software development and operations.
Driven by insatiable curiosity, Noah stays at the forefront of technological advancements, exploring emerging trends in Operations and DevOps. Through engaging publications, he empowers professionals to navigate the complexities of development operations with confidence.
With experience, passion, and a commitment to excellence, Noah is a trusted voice in the Operations and DevOps community. Dedicated to unlocking the potential of this dynamic field, he inspires others to embrace its transformative power.