The world of software development is constantly evolving, and one of the most recent and exciting innovations is Docker. Docker is a containerization technology that simplifies the process of deploying and managing applications in various environments.
With Docker, developers can create isolated environments called containers that bundle an application and all its dependencies together. This approach enables organizations to streamline their development workflows, reduce dependencies on specific operating systems or hardware, and improve portability and scalability. In short, Docker simplifies the process of deploying complex applications and makes it more efficient.
Key Takeaways
- Docker is a containerization technology that simplifies the process of deploying and managing applications
- Docker enables developers to create isolated environments called containers that bundle an application and all its dependencies
- Docker improves the portability and scalability of applications, making them more efficient to manage and maintain
Understanding Container Technology
Container technology revolutionizes how we develop and deploy software, with Docker leading this change. Unlike traditional virtualization, Docker’s containerization speeds up deployment, enhances portability, and boosts efficiency.
Central to Docker’s innovation is its capability to bundle an application’s code and dependencies into a single container. These containers operate in isolation while sharing the host OS’s kernel. This allows multiple containers to coexist on a single host, making application deployment lightweight and flexible.
But the advantages of containerization go beyond just speed and efficiency. Containers ensure consistent environments across development, testing, and production, minimizing error risks. They also offer easy scalability, adapting to the application’s needs.
Moreover, containerization enables modular deployment of application components. This allows developers to focus on smaller, more manageable code segments, simplifying both testing and debugging since each component can be isolated.
Understanding the distinct advantages of Docker’s containerization is crucial for using it to its full potential.
Getting Started with Docker
If you’re new to Docker, the initial setup process may seem daunting. However, with the right guidance, getting started with Docker can be a straightforward process that brings significant benefits to your container deployment workflow.
The first step is to install Docker on your system. Docker provides comprehensive installation guides for Windows, macOS, and Linux systems, which you can access on their website. Once installed, you should verify that Docker is up and running by running the following command in your terminal or command prompt:
docker version
This command will output information about your Docker installation, including the version number and build details.
With Docker installed and running, you can start deploying containers. The first step is to pull a Docker image from a registry. Docker Hub is a popular public registry that hosts thousands of images for various applications. If your application is available as a Docker image on Docker Hub, you can pull it using the following command:
docker pull <image-name>
Replace <image-name> with the name of the Docker image you want to pull. Once the image is downloaded, you can start a container using the following command:
docker run <container-name>
Replace <container-name> with a name of your choice for the container. This command will start a new container based on the image you pulled, and you can verify that it’s running using the following command:
docker ps
This command will output information about your running containers, including the container ID, name, and status.
These are just the basics of getting started with Docker. As you become more familiar with the tool, you can explore more advanced features, such as Docker networking, volumes, and container orchestration with Docker Swarm.
Docker Architecture
Docker architecture is a client-server application with three main components:
- Docker Daemon: This is a background service running on the host operating system, responsible for building, running, and managing Docker containers.
- Docker Client: The Docker client is a command-line tool used by users to interact with the Docker daemon, creating and managing containers.
- Docker Registry: The Docker registry is a repository for Docker images, allowing users to store and share their created or downloaded Docker images with others. Docker Hub is the default public registry provided by Docker.
The Docker architecture follows a client-server model, where the Docker client talks to the Docker daemon via a REST API. And the Docker daemon is responsible for managing the containers, images, and networks. While the Docker registry holds and distributes images, which can be either publicly or privately available.
Docker architecture makes use of the concept of images and containers. A Docker image is a read-only template used to create one or more containers. Docker containers are lightweight, standalone, and executable packages that include everything needed to run an application, including code, libraries, system tools, and runtime. Containers are deployed from images and can be run on any platform that supports Docker.
The Docker architecture enables easy container deployment by abstracting system resources and providing a flexible, isolated environment for running applications. Docker images and containers can be easily versioned, shared, and distributed, making it easy to scale applications to meet changing demand.
Docker Images, Containers, and Registries
A Dockerfile, a text file with a set of build instructions, creates Docker Images. You can build these images using various tools and languages like Bash, Python, and Java.
Docker Containers are runnable instances of Docker Images, compatible with any Docker-enabled host machine. Use the Docker CLI to start, stop, and delete containers.
Docker Registries serve as image repositories, facilitating the storage, distribution, and management of images. Docker Hub is Docker’s default public registry, but other options like Google Container Registry and Amazon Elastic Container Registry are also available. Organizations can establish their own private Docker registries for secure image sharing within their network.
Dockerfile: Building Custom Images
One of the key benefits of Docker is its ability to create custom images that contain your application and all its dependencies. Docker provides a tool called Dockerfile, which is a text file that contains instructions for building a Docker image.
Dockerfile is a simple and easy-to-use tool that allows you to automate the creation of Docker images. You can create a Dockerfile using a text editor or an integrated development environment (IDE) of your choice. Once you have written the Dockerfile, you can use the docker build command to build the image.
Dockerfile Syntax
A Dockerfile consists of a set of instructions that are executed in order to build a Docker image. Each instruction is a keyword followed by arguments. Here is an example of a Dockerfile:
FROM ubuntu:latest RUN apt-get update && apt-get install -y python3-pip COPY . /app WORKDIR /app RUN pip3 install -r requirements.txt EXPOSE 8080 CMD ["python3", "app.py"]
The FROM
instruction specifies the base image to use for the Docker image. The RUN
instruction runs a command in the Docker container. The COPY
instruction copies files from the current directory to the Docker container. The WORKDIR
instruction sets the working directory in the Docker container. The EXPOSE
instruction exposes a port in the Docker container. The CMD
instruction specifies the command to run when the Docker container starts.
Building a Docker Image
Once you have written your Dockerfile, you can use the docker build
command to build the Docker image. Here is an example:
docker build -t myimage .
The -t
option specifies the name and tag for the Docker image. The .
specifies the build context, which is the path to the directory containing the Dockerfile and any other files needed to build the image.
Once the Docker image has been built, you can use the docker run
command to run the container based on the image:
docker run -p 8080:8080 myimage
The -p
option specifies the port mapping between the host and the container. In this example, port 8080 on the host is mapped to port 8080 in the container. The myimage
argument specifies the name of the Docker image to use.
Dockerfile is a powerful tool for creating custom Docker images. By automating the creation of Docker images, you can save time and ensure that your application is built consistently every time. With Dockerfile, you can easily create and deploy custom Docker images that meet the specific needs of your application.
Container Orchestration with Docker Swarm
Docker Swarm serves as Docker’s native solution for clustering and orchestration, streamlining the management of multiple Docker hosts and large-scale container deployment. It automates container orchestration, which includes the deployment, scaling, and networking of containers. Docker Swarm offers a unified entity for managing and deploying containers across various hosts.
In a Docker Swarm setup, you’ll encounter two kinds of nodes: manager nodes and worker nodes. Manager nodes handle control plane operations such as maintaining cluster state, scheduling services, and orchestrating container deployment. Worker nodes execute the actual services and the containers that make up those services.
The architecture of Docker Swarm centers around services, collections of containers performing identical tasks. You can scale a service up or down, and Docker Swarm will automatically distribute the containers among available worker nodes. To deploy a service, create a Docker Compose file that outlines the service configuration, including the number of replicas and the container image to use.
A standout feature of Docker Swarm is its built-in load balancing, which evenly distributes requests among containers running a service. It also offers automatic service discovery, allowing containers on different hosts to communicate seamlessly.
Docker Swarm also supports rolling updates, enabling you to update services without downtime by incrementally replacing old containers with new ones. This ensures service availability during updates.
Docker Swarm eases the management of containerized applications and excels in deploying and scaling them. With features like built-in load balancing and automatic service discovery, it’s an optimal choice for organizations aiming to orchestrate Docker containers at scale.
Docker Networking
Docker offers robust networking capabilities for container-to-container and container-to-external-world communication. By default, Docker sets up a bridge network for intra-host container communication. However, it also supports various network drivers for different networking types.
To establish a custom network, execute the docker network create command. This action creates a new network to which containers can connect, using the bridge driver by default. Alternative drivers like host and overlay are also available for diverse networking needs.
Use the docker network connect command to link a container to a specific network, enabling it to interact with other containers on that network. To disconnect a container, employ the docker network disconnect command.
Docker also allows port exposure to external traffic using the -p option, mapping a container port to a host system port. The –link option facilitates container linking, enabling inter-container communication over the Docker network.
Docker’s networking features offer a versatile framework for configuring and managing container communication. By grasping Docker’s networking functionalities, you can construct intricate container networks for building highly scalable and adaptable applications.
Docker Volumes: Data Persistence
Data persistence is a crucial aspect of container deployment, and Docker volumes make it easier to manage data across containers. Docker volumes are directories stored outside the container’s filesystem, allowing data to persist even after a container has been deleted or recreated.
When creating a volume, Docker ensures that it is stored on the host machine and can be accessed by the container. Alternatively, volumes can be created using a specific driver, such as the Amazon EBS driver, which enables volumes to be stored on Amazon Elastic Block Store.
Docker volumes can be managed using the Docker CLI or Docker Compose. Volumes can be created and listed using the following commands:
docker volume create <volume_name> docker volume ls
Volumes can also be mounted within containers. When starting a container, the docker run command can be used to mount a volume:
docker run -v <volume_name>:<container_path> <image_name>
This creates a new container using the specified image and mounts the volume at the container path specified. Any data written to that path is stored in the volume.
Using volumes, data can be shared between multiple containers. This is useful for scenarios such as running a stateful database in one container and a web application in another. By mounting the same volume in both containers, they can share data stored in that volume.
Overall, Docker volumes provide a convenient way to manage data persistence in containerized environments, improving application performance and reliability. Understanding how to create and manage volumes is essential for efficient container deployment.
Docker Security Best Practices
As with any technology, security is a top concern when using Docker for container deployment. By following best practices, you can minimize vulnerabilities and protect your applications and data.
Understand Docker Security Risks
Before diving into best practices, it’s important to understand the potential security risks associated with using Docker. These include malicious images, unsecured APIs, and vulnerabilities in container runtimes. By being aware of these risks, you can take proactive steps to prevent them.
Follow Container Image Best Practices
One of the easiest ways to improve container security is to use trusted images from verified sources. When creating custom images, be sure to update all packages and avoid adding unnecessary software or dependencies. Limiting user privileges within containers can also help prevent malicious activity.
Secure Your Docker Environment
Securing the underlying Docker environment is vital to protecting containers. This includes limiting network exposure, implementing strong authentication and access controls, and regularly updating Docker components to address vulnerabilities.
Use Docker Bench Security
Docker Bench Security is a great tool to use for automated security checks on your Docker deployment. It can help identify potential vulnerabilities and guide you in implementing security best practices.
Monitor Container Activity
Monitoring container activity can help identify any suspicious behavior or activity. Tools such as Sysdig and Docker Security Scanning can help detect vulnerabilities and provide insights into container activity.
Regularly Perform Security Audits
Regularly audit your Docker environment for potential security risks. This includes checking for outdated software, unused containers or images, and any misconfigured settings.
By following these best practices, you can help ensure the security of your Docker deployment and protect your applications and data from potential threats.
Monitoring and Debugging with Docker
Monitoring and debugging are critical activities in ensuring the optimal performance of any application deployed using Docker. While Docker provides a level of transparency into the application’s inner workings, additional monitoring and debugging techniques are necessary to identify bottlenecks and errors.
Monitoring Docker Applications
One of the most popular tools for monitoring Docker applications is Prometheus, an open-source monitoring system that provides real-time metrics and a graphical user interface for analysis. Prometheus can be easily integrated with Docker containers, allowing for seamless monitoring of individual containers and their respective applications.
Another popular monitoring tool is Grafana, which can be used in conjunction with Prometheus to visualize and alert on metrics. Grafana supports multiple data sources, allowing for the integration of additional monitoring tools such as Nagios and Zabbix.
Debugging Docker Applications
Debugging Docker applications can be challenging due to the containerized nature of the applications. However, several tools are available to make debugging easier.
One such tool is Docker Compose, which enables the creation of multi-container environments for testing and debugging. Compose creates a single Docker network for all containers, making it easier to connect and debug each container in the environment.
The Docker logs command is another useful debugging tool, providing real-time access to container logs. This is particularly helpful in identifying errors and troubleshooting issues that may arise during container runtime.
Monitoring and debugging are critical activities when deploying applications using Docker. With the right monitoring and debugging tools, developers can gain invaluable insights into application performance and quickly identify issues that may be impacting performance. By incorporating these techniques into development workflows, developers can streamline application deployment and ensure optimal performance for end-users.
Future Trends in Containerization
Containerization is a rapidly evolving technology that has already disrupted the software industry. It has transformed how organizations design, build, ship, and manage software applications. As we look towards the future, there are several emerging trends that are likely to shape the containerization landscape.
Kubernetes
Kubernetes is an open-source platform that automates container operations. It provides a scalable and resilient infrastructure for deploying and managing containerized applications. Kubernetes is rapidly becoming the de facto standard for container orchestration, and its adoption is expected to continue to increase in the coming years.
Serverless Architecture
Serverless architecture is a cloud computing model where the cloud provider manages the infrastructure and automatically provisions resources as needed. It allows developers to focus on writing code without worrying about the underlying infrastructure. Containerization and serverless architecture are complementary technologies, and the use of containers in serverless computing is a trend that is likely to gain traction.
Broader Adoption of Container Technology
The adoption of container technology has grown rapidly in recent years, but it is still not mainstream. As containerization matures and becomes easier to use, we can expect to see broader adoption across industries and applications. The benefits of containers, such as improved portability, scalability, and efficiency, will drive their wider adoption.
FAQ
Q: What is Docker containerization?
A: Docker containerization is a method of packaging software applications along with their dependencies into a standardized unit called a container. Containers provide a lightweight and isolated environment that can run consistently across different operating systems and infrastructures.
Q: How does Docker work?
A: Docker uses the concept of containers to package and isolate applications. Each container runs as an isolated process, with its own file system, network interface, and process space. Docker containers share the host system’s operating system (OS) kernel, which makes them more lightweight and efficient than traditional virtual machines.
Q: What is a Docker image?
A: A Docker image is a read-only template that contains a set of instructions for creating a Docker container. It includes the application code, runtime environment, libraries, and dependencies needed to run the application. Docker images are stored in a Docker registry and can be pulled and run on different systems.
Q: What is a Docker registry?
A: A Docker registry is a centralized repository for storing and distributing Docker images. It acts as a storage location for Docker images and allows users to push and pull images from different environments. Docker Hub is the default public registry, but users can also set up their private registries.
Q: How do I use Docker containers?
A: To use Docker containers, you need to follow these steps: 1. Install Docker on your system. 2. Pull or build a Docker image. 3. Run a Docker container using the image. 4. Interact with the running container (e.g., accessing a web server running inside the container). 5. Stop or remove the container when you’re done.
Q: Is Docker only for Linux?
A: Docker was initially developed for Linux, but it now supports multiple operating systems, including Windows and macOS. Docker uses OS-level virtualization technology to run containers, which allows it to work on different operating systems.
Q: What is the difference between a Docker container and a virtual machine?
A: The main difference between a Docker container and a virtual machine (VM) is the level of isolation. VMs provide full isolation by running a separate operating system on top of the host system’s OS. Containers, on the other hand, share the host system’s OS kernel, making them more lightweight and faster to start compared to VMs.
Q: How can Docker benefit DevOps engineers?
A: Docker simplifies the deployment and management of applications, making it easier for DevOps engineers to package, ship, and run applications across different environments. Docker containers provide consistency and reproducibility, speeding up the development and testing process. They also enable the scaling of applications and microservices architectures.
Q: What are the next steps after learning Docker?
A: After learning Docker, you might consider exploring related technologies such as Kubernetes for container orchestration, continuous integration/continuous deployment (CI/CD) tools for automated deployments, and cloud platforms that integrate well with Docker for scalable and fault-tolerant architectures.
Q: Where can I find resources for Docker tutorials and documentation?
A: You can find Docker tutorials and official documentation on the Docker website (docker.com). The Docker documentation provides detailed guides, examples, and best practices for using Docker and related tools.
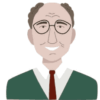
James is an esteemed technical author specializing in Operations, DevOps, and computer security. With a master’s degree in Computer Science from CalTech, he possesses a solid educational foundation that fuels his extensive knowledge and expertise. Residing in Austin, Texas, James thrives in the vibrant tech community, utilizing his cozy home office to craft informative and insightful content. His passion for travel takes him to Mexico, a favorite destination where he finds inspiration amidst captivating beauty and rich culture. Accompanying James on his adventures is his faithful companion, Guber, who brings joy and a welcome break from the writing process on long walks.
With a keen eye for detail and a commitment to staying at the forefront of industry trends, James continually expands his knowledge in Operations, DevOps, and security. Through his comprehensive technical publications, he empowers professionals with practical guidance and strategies, equipping them to navigate the complex world of software development and security. James’s academic background, passion for travel, and loyal companionship make him a trusted authority, inspiring confidence in the ever-evolving realm of technology.