Kubernetes has emerged as the leading open-source platform for container orchestration. It revolutionizes the way applications are deployed, scaled, and managed in modern software development.
Mastering Kubernetes is essential for developers and DevOps engineers looking to stay competitive in today’s rapidly evolving tech landscape. But mastery isn’t just about reading documentation. It’s about diving deep into practical applications and real-world scenarios.
Understanding Kubernetes Basics
Kubernetes, often abbreviated as K8s, automates the deployment, scaling, and management of containerized applications. It provides a robust framework for orchestrating containers across clusters of machines. This makes it easier to build and run distributed systems.
Core Components and Concepts
Understanding the core components and concepts of Kubernetes is crucial. Let’s break them down with examples and explanations.
Pods: The Smallest Deployable Units
Pods are the smallest deployable units in Kubernetes. They can contain one or more containers.
Example: Simple Pod Definition
apiVersion: v1
kind: Pod
metadata:
name: nginx-pod
spec:
containers:
- name: nginx
image: nginx:latest
ports:
- containerPort: 80
Explanation:
apiVersion
,kind
, andmetadata
: Define the Pod’s API version, type, and metadata.spec
: Specifies the Pod’s desired state.containers
: List of containers in the Pod. Here, it’s a single nginx container.
Diagram: Pod Structure
Pod
└── Container: nginx
Deployments: Manage the Lifecycle of Applications
Deployments manage the lifecycle of your applications. They ensure the desired state of the application is maintained.
Example: Deployment YAML
apiVersion: apps/v1
kind: Deployment
metadata:
name: webapp-deployment
spec:
replicas: 3
selector:
matchLabels:
app: webapp
template:
metadata:
labels:
app: webapp
spec:
containers:
- name: webapp
image: webapp:1.0
ports:
- containerPort: 80
Explanation:
replicas
: Number of Pod replicas to run.selector
: Identifies the Pods managed by the Deployment.template
: Pod template used to create Pods.
Diagram: Deployment Structure
Deployment: webapp-deployment
├── ReplicaSet
│ └── Pod: webapp (3 replicas)
Services: Expose Applications to the Network
Services expose your applications to the network. They provide stable IPs and DNS names.
Example: Service YAML
apiVersion: v1
kind: Service
metadata:
name: webapp-service
spec:
selector:
app: webapp
ports:
- protocol: TCP
port: 80
targetPort: 80
type: LoadBalancer
Explanation:
selector
: Matches Pods with the labelapp: webapp
.ports
: Specifies the service port and target port.type
:LoadBalancer
exposes the service externally.
Diagram: Service Structure
Service: webapp-service
└── Pods: webapp (matched by selector)
ConfigMaps and Secrets: Manage Configuration Data
ConfigMaps and Secrets store configuration data and sensitive information, respectively.
Example: ConfigMap YAML
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
DATABASE_URL: "localhost:5432/mydb"
Example: Secret YAML
apiVersion: v1
kind: Secret
metadata:
name: app-secret
type: Opaque
data:
password: cGFzc3dvcmQ=
Explanation:
ConfigMap
: Stores configuration data likeDATABASE_URL
.Secret
: Stores sensitive data like passwords, encoded in base64.
Diagram: ConfigMap and Secret Structure
ConfigMap: app-config
└── DATABASE_URL
Secret: app-secret
└── password
Volumes: Provide Persistent Storage
Volumes provide storage that persists beyond the life of individual Pods.
Example: Volume and PersistentVolumeClaim
apiVersion: v1
kind: PersistentVolume
metadata:
name: pv
spec:
capacity:
storage: 1Gi
accessModes:
- ReadWriteOnce
hostPath:
path: /data/pv
PersistentVolumeClaim
apiVersion: v1
kind: PersistentVolumeClaim
metadata:
name: pvc
spec:
accessModes:
- ReadWriteOnce
resources:
requests:
storage: 1Gi
Explanation:
PersistentVolume
: Defines storage available to the cluster.PersistentVolumeClaim
: Requests storage defined by thePersistentVolume
.
Diagram: Volume Structure
PersistentVolume: pv
└── Storage: /data/pv
PersistentVolumeClaim: pvc
└── Requests: 1Gi
Service Discovery: Locate Services Within the Cluster
Service Discovery helps locate services within the cluster using DNS.
Example: Service with DNS
apiVersion: v1
kind: Service
metadata:
name: backend-service
spec:
selector:
app: backend
ports:
- protocol: TCP
port: 80
targetPort: 8080
Explanation:
- The service
backend-service
can be accessed by other Pods using DNS namebackend-service
.
Diagram: Service Discovery Structure
Service: backend-service
└── DNS: backend-service
RBAC: Manage Access to Cluster Resources
RBAC (Role-Based Access Control) manages who can do what within the cluster.
Example: RBAC YAML
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
RoleBinding
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: default
subjects:
- kind: User
name: jane
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
Explanation:
Role
: Defines permissions, like reading Pods.RoleBinding
: Assigns the role to a user, in this case,jane
.
Diagram: RBAC Structure
Role: pod-reader
└── Permissions: get, watch, list Pods
RoleBinding: read-pods
└── User: jane
Mastering these core components and concepts is essential for effective Kubernetes use. They form the foundation for more advanced operations and optimizations. Dive deep, practice, and explore real-world applications to solidify your understanding.
Practical Applications
To master Kubernetes, focus on practical applications. Theoretical knowledge is a foundation, but hands-on practice is where real understanding develops. Many resources emphasize real-world scenarios, such as deploying full-stack applications using a microservices architecture.
Deploying a Simple Web Application
Let’s walk through deploying a simple web application in Kubernetes. We’ll create a deployment, expose it via a service, and then scale it to handle more load.
Step 1: Create a Deployment
First, create a deployment using a YAML file. This file defines the application’s deployment configuration.
Example: Deployment YAML
apiVersion: apps/v1
kind: Deployment
metadata:
name: webapp-deployment
spec:
replicas: 3
selector:
matchLabels:
app: webapp
template:
metadata:
labels:
app: webapp
spec:
containers:
- name: webapp
image: webapp:1.0
ports:
- containerPort: 80
Explanation:
apiVersion
andkind
: Specify this is a deployment configuration.metadata
: Includes the deployment name.spec
: Details the deployment specifications.replicas
: Sets the number of pod replicas to create (3 in this case).selector
: Matches the pods with the labelapp: webapp
.template
: Defines the pod template, including metadata and spec.containers
: Lists the container specifications. Here, it’s a single container namedwebapp
using thewebapp:1.0
image and exposing port 80.
Diagram: Deployment Structure
Deployment: webapp-deployment
├── ReplicaSet
│ └── Pods: webapp (3 replicas)
Step 2: Expose the Deployment
Next, create a service to expose the deployment. This service makes the web application accessible from the network.
Example: Service YAML
apiVersion: v1
kind: Service
metadata:
name: webapp-service
spec:
selector:
app: webapp
ports:
- protocol: TCP
port: 80
targetPort: 80
type: LoadBalancer
Explanation:
apiVersion
andkind
: Specify this is a service configuration.metadata
: Includes the service name.spec
: Details the service specifications.selector
: Matches pods with the labelapp: webapp
.ports
: Specifies the service port (80) and the target port (80).type
: Set toLoadBalancer
to expose the service externally.
Diagram: Service Structure
Service: webapp-service
└── Pods: webapp (matched by selector)
Step 3: Scale Your Application
Adjust the number of replicas to manage the application’s load. Scaling helps handle increased traffic or workload.
Example: Scaling Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: webapp-deployment
spec:
replicas: 10 # Adjusted from 3 to 10
selector:
matchLabels:
app: webapp
template:
metadata:
labels:
app: webapp
spec:
containers:
- name: webapp
image: webapp:1.0
ports:
- containerPort: 80
Explanation:
replicas
: Updated from 3 to 10 to scale the application and handle more traffic.
Diagram: Scaled Deployment Structure
Deployment: webapp-deployment
├── ReplicaSet
│ └── Pods: webapp (10 replicas)
This hands-on practice is key to mastering Kubernetes and understanding its real-world applications.
Advanced Topics
As you progress in your Kubernetes journey, delve into advanced topics. These areas will help you optimize your cluster, enhance security, monitor performance, ensure high availability, and extend functionality.
Cluster Management and Optimization
Efficiently managing resources is crucial for a well-functioning cluster. This includes configuring resource requests and limits to prevent resource contention.
Example: Resource Requests and Limits
apiVersion: v1
kind: Pod
metadata:
name: optimized-pod
spec:
containers:
- name: app
image: app:latest
resources:
requests:
memory: "64Mi"
cpu: "250m"
limits:
memory: "128Mi"
cpu: "500m"
Explanation:
requests
: Minimum resources required.limits
: Maximum resources allowed.
Diagram: Resource Management
Pod: optimized-pod
└── Container: app
├── Requests: 64Mi memory, 250m CPU
└── Limits: 128Mi memory, 500m CPU
Security Best Practices
Protect your cluster and applications by implementing security best practices like Role-Based Access Control (RBAC) and network policies.
Example: Network Policy
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-only-frontend
spec:
podSelector:
matchLabels:
role: backend
ingress:
- from:
- podSelector:
matchLabels:
role: frontend
Explanation:
podSelector
: Selects the backend pods.ingress
: Allows traffic only from pods with the labelrole: frontend
.
Diagram: Network Policy
└── Ingress: Allow from pods with label role=frontend
Monitoring and Logging
Gain insights into cluster performance using monitoring and logging tools like Prometheus and Grafana.
Example: Prometheus Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: prometheus
spec:
replicas: 1
selector:
matchLabels:
app: prometheus
template:
metadata:
labels:
app: prometheus
spec:
containers:
- name: prometheus
image: prom/prometheus
ports:
- containerPort: 9090
Explanation:
- Deploys Prometheus to monitor the cluster.
Diagram: Monitoring Setup
Deployment: prometheus
└── Pod: prometheus
└── Container: prom/prometheus (port 9090)
High Availability and Disaster Recovery
Ensure uptime and data integrity by configuring high availability and disaster recovery strategies.
Example: StatefulSet for High Availability
apiVersion: apps/v1
kind: StatefulSet
metadata:
name: webapp
spec:
serviceName: "webapp"
replicas: 3
selector:
matchLabels:
app: webapp
template:
metadata:
labels:
app: webapp
spec:
containers:
- name: webapp
image: webapp:1.0
ports:
- containerPort: 80
Explanation:
StatefulSet
: Ensures the order and uniqueness of pods.
Diagram: StatefulSet Structure
PersistentVolume: pv
└── Storage: /data/pv
PersistentVolumeClaim: pvc
└── Requests: 1Gi
Service Discovery: Locate Services Within the Cluster
Service Discovery helps locate services within the cluster using DNS.
Example: Service with DNS
apiVersion: v1
kind: Service
metadata:
name: backend-service
spec:
selector:
app: backend
ports:
- protocol: TCP
port: 80
targetPort: 8080
Explanation:
StatefulSet
: Ensures the order and uniqueness of pods.
Diagram: StatefulSet Structure
StatefulSet: webapp
├── Pod: webapp-0
├── Pod: webapp-1
└── Pod: webapp-2
Custom Resource Definitions (CRDs) and Operators
Extend Kubernetes functionality with Custom Resource Definitions (CRDs) and Operators.
Example: Custom Resource Definition
apiVersion: apiextensions.k8s.io/v1
kind: CustomResourceDefinition
metadata:
name: crontabs.stable.example.com
spec:
group: stable.example.com
versions:
- name: v1
served: true
storage: true
schema:
openAPIV3Schema:
type: object
properties:
spec:
type: object
properties:
cronSpec:
type: string
image:
type: string
replicas:
type: integer
scope: Namespaced
names:
plural: crontabs
singular: crontab
kind: CronTab
shortNames:
- ct
Explanation:
CustomResourceDefinition
: Defines a new resource typeCronTab
.
Diagram: CRD Structure
Service: backend-service
└── DNS: backend-service
These advanced topics help you fine-tune your Kubernetes skills, ensuring you can manage resources efficiently, secure your cluster, monitor performance, maintain high availability, and extend Kubernetes functionality. Mastering these areas is essential for leveraging the full power of Kubernetes in real-world applications.
Continuous Learning and Practice
Mastering Kubernetes is an ongoing process. Technology evolves, and so should your skills. Stay updated with the latest Kubernetes releases and best practices. Join communities, participate in forums, and keep learning.
Recommended Resources
- Books: “Mastering Kubernetes” by Packt Publishing.
- Courses: AmigosCode offers comprehensive Kubernetes courses.
- Communities: Kubernetes Slack, Stack Overflow, and GitHub discussions.
Real-World Challenges
Theoretical knowledge alone won’t cut it. You must understand the complexities of real-world applications. Let’s delve into some common challenges you might face.
Resource Management
Efficiently managing resources is crucial to avoid over-provisioning or under-provisioning.
Example: Resource Quotas
apiVersion: v1
kind: ResourceQuota
metadata:
name: compute-resources
spec:
hard:
requests.cpu: "4"
requests.memory: "8Gi"
limits.cpu: "10"
limits.memory: "16Gi"
Explanation:
ResourceQuota
: Limits the total amount of resources (CPU and memory) that can be used in a namespace.
Diagram: Resource Quota
ResourceQuota: compute-resources
├── Requests: 4 CPU, 8Gi memory
└── Limits: 10 CPU, 16Gi memory
Scaling
Handling sudden spikes in traffic and ensuring high availability is critical.
Example: Horizontal Pod Autoscaler
apiVersion: autoscaling/v1
kind: HorizontalPodAutoscaler
metadata:
name: webapp-autoscaler
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: webapp-deployment
minReplicas: 2
maxReplicas: 10
targetCPUUtilizationPercentage: 50
Explanation:
HorizontalPodAutoscaler
: Automatically scales the number of pod replicas based on CPU utilization.
Diagram: Autoscaler
HorizontalPodAutoscaler: webapp-autoscaler
├── Target: webapp-deployment
├── MinReplicas: 2
└── MaxReplicas: 10
Security
Implementing RBAC, network policies, and secret management to secure your cluster.
Example: RBAC Role and RoleBinding
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "list", "watch"]
RoleBinding
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: default
subjects:
- kind: User
name: jane
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
Explanation:
Role
: Grants read access to pods.RoleBinding
: Assigns the role to userjane
.
Diagram: RBAC
Role: pod-reader
├── Permissions: get, list, watch pods
RoleBinding: read-pods
└── User: jane
Persistence
Managing data persistence and backups to ensure data integrity.
Example: PersistentVolume and PersistentVolumeClaim
apiVersion: v1
kind: PersistentVolume
metadata:
name: pv
spec:
capacity:
storage: 5Gi
accessModes:
- ReadWriteOnce
hostPath:
path: "/data/pv"
PersistentVolumeClaim
apiVersion: v1
kind: PersistentVolumeClaim
metadata:
name: pvc
spec:
accessModes:
- ReadWriteOnce
resources:
requests:
storage: 5Gi
Explanation:
PersistentVolume
: Defines the storage available.PersistentVolumeClaim
: Requests the storage.
Diagram: Persistence
PersistentVolume: pv
└── Storage: /data/pv
PersistentVolumeClaim: pvc
└── Requests: 5Gi storage
Networking
Configuring services, ingress controllers, and load balancers to manage traffic.
Example: Ingress Resource
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: webapp-ingress
spec:
rules:
- host: webapp.example.com
http:
paths:
- path: /
pathType: Prefix
backend:
service:
name: webapp-service
port:
number: 80
Explanation:
Ingress
: Manages external access to services, providing HTTP and HTTPS routing.
Diagram: Ingress
Ingress: webapp-ingress
└── Host: webapp.example.com
└── Path: /
└── Backend: webapp-service (port 80)
Mastering these real-world challenges is essential for efficient and effective Kubernetes management. Focus on resource management, scaling, security, persistence, and networking to ensure your applications run smoothly and securely.
Wrapping up
Mastering Kubernetes requires a blend of fundamental knowledge, hands-on practice, and real-world experience. It’s not just about reading the documentation but applying it to solve complex problems. Kubernetes is constantly evolving, and so should your skills. Stay curious, keep learning, and engage with the community. As you gain proficiency, you’ll be better equipped to design, implement, and manage large-scale distributed applications efficiently.
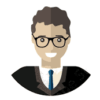
Noah is an accomplished technical author specializing in Operations and DevOps, driven by a passion ignited during his tenure at eBay in 2000. With over two decades of experience, Noah shares his transformative knowledge and insights with the community.
Residing in a charming London townhouse, he finds inspiration in the vibrant energy of the city. From his cozy writing den, overlooking bustling streets, Noah immerses himself in the evolving landscape of software development, operations, and technology. Noah’s impressive professional journey includes key roles at IBM and Microsoft, enriching his understanding of software development and operations.
Driven by insatiable curiosity, Noah stays at the forefront of technological advancements, exploring emerging trends in Operations and DevOps. Through engaging publications, he empowers professionals to navigate the complexities of development operations with confidence.
With experience, passion, and a commitment to excellence, Noah is a trusted voice in the Operations and DevOps community. Dedicated to unlocking the potential of this dynamic field, he inspires others to embrace its transformative power.