Scaling a SaaS application in the world of DevOps is no small feat. Imagine trying to build a skyscraper on an unsteady foundation. The complexities involved can easily cause teams to miss critical elements. This often results in unstable services, overshadowing the fundamentals of growth. Ultimately, this can lead to customer dissatisfaction.
From my experience navigating this journey multiple times, I’ve gathered valuable insights. These lessons can help you scale your SaaS while maintaining quality and reliability. Let’s explore how.
Understanding the DevOps Environment
The Duality of DevOps
DevOps combines development and operations. This integration aims to improve collaboration and productivity by automating infrastructure, workflows, and continuous measurement of application performance. However, this duality can sometimes create a complex environment where scaling becomes challenging.
Common Pitfalls
Teams often face several common pitfalls. Overemphasis on speed can lead to unstable deployments. Ignoring automation results in manual errors. Failing to monitor performance causes unseen issues to fester. Addressing these pitfalls is crucial for successful scaling.
Laying a Strong Foundation
Emphasize Automation
Automation is the bedrock of scaling in DevOps. By automating repetitive tasks, you reduce the risk of human error and free up your team to focus on more strategic activities. Tools like Jenkins, Ansible, and Terraform can streamline your CI/CD pipeline, infrastructure provisioning, and configuration management.
# Example of a simple Ansible playbook for deploying a web server
- hosts: webservers
tasks:
- name: Install Apache
apt:
name: apache2
state: present
- name: Start Apache
service:
name: apache2
state: started
Prioritize Continuous Integration and Continuous Deployment (CI/CD)
CI/CD practices ensure that code changes are automatically tested and deployed. This reduces the time between development and deployment, leading to faster iterations and more stable releases. Integrating CI/CD pipelines using tools like GitLab CI, CircleCI, or Jenkins can make a significant difference.
# Example GitLab CI pipeline configuration
stages:
- build
- test
- deploy
build:
stage: build
script:
- echo "Building the application..."
test:
stage: test
script:
- echo "Running tests..."
deploy:
stage: deploy
script:
- echo "Deploying to production..."
Ensuring Robust Monitoring and Logging
Implement Comprehensive Monitoring
Monitoring is essential for understanding the health and performance of your application. Use tools like Prometheus, Grafana, and New Relic to monitor metrics such as CPU usage, memory consumption, and request latency. This data helps identify issues before they impact users.
Importance of Logging
Logging provides insight into the application’s behavior and is crucial for troubleshooting. Implement centralized logging using tools like ELK Stack (Elasticsearch, Logstash, and Kibana) or Splunk. Ensure that logs are structured and searchable.
{
"timestamp": "2023-06-19T12:34:56Z",
"level": "ERROR",
"message": "Failed to connect to database",
"service": "user-service",
"trace": "Stack trace here"
}
Scalability Through Microservices
Benefits of Microservices Architecture
Microservices allow you to break down your application into smaller, independent services. This makes scaling easier because you can scale each service independently based on its needs. It also improves fault isolation, as failures in one service do not affect the entire system.
Challenges and Solutions
Microservices come with their own set of challenges, such as inter-service communication and data consistency. Use tools like Kubernetes for container orchestration and service meshes like Istio to manage communication between services. Adopt patterns like Saga for managing distributed transactions.
Fostering a DevOps Culture
Encourage Collaboration
A successful DevOps environment thrives on collaboration. Break down silos between development and operations teams. Encourage open communication and knowledge sharing. Use collaboration tools like Slack, Jira, and Confluence to facilitate this.
Invest in Training
Continuous learning is vital. Invest in training your team on the latest DevOps practices and tools. Certifications in AWS, Azure, and Kubernetes can provide valuable knowledge and skills. Encourage attendance at DevOps conferences and meetups.
Leveraging Cloud Services
Choose the Right Cloud Provider
Cloud services offer scalable infrastructure without the overhead of managing physical servers. Providers like AWS, Azure, and Google Cloud offer various services tailored to different needs. Evaluate their offerings and choose the one that best fits your requirements.
Optimize for Cost and Performance
Cloud resources can quickly become expensive if not managed properly. Use auto-scaling to adjust resources based on demand. Implement cost monitoring and optimization tools like AWS Cost Explorer or Google Cloud’s Cost Management.
Security
Integrate Security into DevOps
Security should be an integral part of your DevOps process. Implement DevSecOps practices to integrate security at every stage of development. Use tools like Snyk and Aqua Security to scan for vulnerabilities in your code and containers.
Regular Audits and Compliance
Regular security audits help identify and mitigate risks. Ensure compliance with industry standards like GDPR, HIPAA, and SOC 2. Use automated compliance tools to monitor and enforce compliance continuously.
Example
Here’s an hypothetical SaaS company, “ScaleUp Solutions,” which successfully scaled its application using DevOps practices. Initially, ScaleUp Solutions faced several challenges, including slow deployment speeds and unstable system performance. Here’s how they transformed their processes to achieve scalable and reliable services.
Initial Challenges
Deployment Speed: The company experienced significant delays in deploying new features. Manual processes and lengthy approval chains caused bottlenecks, leading to slow time-to-market.
System Stability: Frequent downtimes and performance issues plagued their application. This instability resulted in customer dissatisfaction and increased support requests.
Transformation Through DevOps
Adopting CI/CD
Problem: Manual deployments were error-prone and time-consuming.
Solution: ScaleUp Solutions implemented Continuous Integration and Continuous Deployment (CI/CD) pipelines to automate the testing and deployment process.
Example: They used Jenkins to set up a CI/CD pipeline.
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building the application...'
sh './build.sh'
}
}
stage('Test') {
steps {
echo 'Running tests...'
sh './test.sh'
}
}
stage('Deploy') {
steps {
echo 'Deploying to production...'
sh './deploy.sh'
}
}
}
post {
always {
archiveArtifacts artifacts: '**/target/*.jar', fingerprint: true
junit 'test-results/**/*.xml'
}
}
}
Outcome: This pipeline enabled automated builds, tests, and deployments, reducing human error and speeding up the release cycle.
Automating Infrastructure
Problem: Manual infrastructure management was inefficient and error-prone.
Solution: They adopted Infrastructure as Code (IaC) using Terraform and Ansible to automate provisioning and configuration.
Example: Using Terraform to provision AWS resources.
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "web-instance"
}
}
Example: Using Ansible to configure the instances.
- hosts: webservers
tasks:
- name: Install Nginx
apt:
name: nginx
state: present
- name: Start Nginx
service:
name: nginx
state: started
Outcome: Automating infrastructure reduced setup time, minimized errors, and ensured consistent environments across development, testing, and production.
Transitioning to Microservices
Problem: The monolithic architecture was hard to scale and maintain.
Solution: They transitioned to a microservices architecture, allowing independent scaling and deployment of services.
Example: Using Docker to containerize services.
# Dockerfile for the user service
FROM openjdk:8-jdk-alpine
VOLUME /tmp
COPY target/user-service.jar user-service.jar
ENTRYPOINT ["java","-jar","/user-service.jar"]
Example: Deploying containers using Kubernetes.
apiVersion: apps/v1
kind: Deployment
metadata:
name: user-service
spec:
replicas: 3
selector:
matchLabels:
app: user-service
template:
metadata:
labels:
app: user-service
spec:
containers:
- name: user-service
image: user-service:latest
ports:
- containerPort: 8080
Outcome: Microservices improved scalability and fault isolation, allowing the team to deploy updates to individual services without affecting the entire system.
Robust Monitoring System
Problem: Lack of visibility into application performance and issues.
Solution: They implemented a comprehensive monitoring system using Prometheus for metrics collection and Grafana for visualization.
Example: Setting up Prometheus for monitoring.
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'prometheus'
static_configs:
- targets: ['localhost:9090']
Example: Creating a Grafana dashboard.
{
"dashboard": {
"panels": [
{
"type": "graph",
"title": "CPU Usage",
"targets": [
{
"expr": "rate(node_cpu_seconds_total[1m])",
"format": "time_series"
}
]
}
]
}
}
Outcome: This setup provided real-time insights into system performance, enabling proactive issue resolution and capacity planning.
Fostering a DevOps Culture
Problem: Siloed teams and lack of collaboration hindered progress.
Solution: They fostered a DevOps culture by encouraging collaboration between development and operations teams, promoting a shared responsibility for system health and performance.
Outcome: Regular cross-functional meetings and shared goals improved communication and teamwork, leading to faster problem resolution and innovation.
Investing in Training
Problem: Knowledge gaps in the latest DevOps tools and practices.
Solution: The company invested in continuous learning by providing training programs, certifications, and opportunities to attend industry conferences.
Outcome: A well-trained team stayed updated with the latest trends and best practices, contributing to improved processes and innovation.
This example demonstrates that with the right approach, scaling a SaaS application in the DevOps world is achievable. By focusing on automation, continuous integration, and fostering a collaborative culture, you can ensure your SaaS scales efficiently and reliably, leading to satisfied customers and sustained growth.
Final Thoughts
Scaling your SaaS in the DevOps world might seem daunting. However, with a solid foundation, automation, and a focus on monitoring, it becomes manageable. Embrace microservices, foster a collaborative culture, and leverage cloud services. Prioritize security and continuous learning.
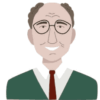
James is an esteemed technical author specializing in Operations, DevOps, and computer security. With a master’s degree in Computer Science from CalTech, he possesses a solid educational foundation that fuels his extensive knowledge and expertise. Residing in Austin, Texas, James thrives in the vibrant tech community, utilizing his cozy home office to craft informative and insightful content. His passion for travel takes him to Mexico, a favorite destination where he finds inspiration amidst captivating beauty and rich culture. Accompanying James on his adventures is his faithful companion, Guber, who brings joy and a welcome break from the writing process on long walks.
With a keen eye for detail and a commitment to staying at the forefront of industry trends, James continually expands his knowledge in Operations, DevOps, and security. Through his comprehensive technical publications, he empowers professionals with practical guidance and strategies, equipping them to navigate the complex world of software development and security. James’s academic background, passion for travel, and loyal companionship make him a trusted authority, inspiring confidence in the ever-evolving realm of technology.