For many teams, CI/CD is more of a mythical beast than a practical tool. People get lost in the tangle of complex definitions without capturing its true essence or realizing its potential. As someone who has implemented CI/CD practices successfully across multiple organizations, I can share an accessible, yet simple, understanding of CI/CD that will change the way your team works.
Demystifying CI/CD
Continuous Integration and Continuous Deployment (CI/CD) can revolutionize how software development teams operate. However, for many teams, CI/CD remains an elusive concept. They get bogged down in jargon and complexity, failing to grasp its true potential. CI/CD is not just a technical process; it’s a mindset and a culture. Implementing CI/CD practices effectively can dramatically improve productivity, code quality, and deployment speed.
The Essence of CI/CD
CI/CD is about automating the integration and deployment processes. Continuous Integration (CI) involves frequently merging code changes into a central repository. Automated builds and tests run on every commit. This ensures that new code integrates smoothly and quickly. Continuous Deployment (CD), on the other hand, is about automating the release of validated builds to production.
By focusing on automation, CI/CD reduces manual intervention. This minimizes errors and speeds up the development cycle. But to truly understand CI/CD, we need to delve into its core principles and practices.
Core Principles of CI/CD
1. Automation
Automation is the backbone of CI/CD. Automated tests, builds, and deployments eliminate repetitive tasks. They ensure consistency and reliability. Tools like Jenkins, CircleCI, and GitLab CI/CD are popular for this purpose. These tools help in setting up pipelines that automate the entire process from code commit to deployment.
2. Collaboration
CI/CD fosters collaboration among team members. Developers, testers, and operations work together seamlessly. This unified approach leads to better communication and fewer misunderstandings. Version control systems like Git play a crucial role here. They facilitate code sharing and collaboration.
3. Continuous Improvement
CI/CD encourages continuous improvement. By regularly integrating and deploying code, teams can quickly identify and fix issues. This leads to faster feedback loops and improved code quality. It also allows teams to respond rapidly to changing requirements and market conditions.
Implementing CI: A Practical Guide
Implementing CI involves setting up automated builds and tests. Here’s a step-by-step guide to get you started:
Step 1: Choose Your Tools
Select a CI tool that fits your team’s needs. Jenkins is a popular choice due to its flexibility and extensive plugin ecosystem. GitLab CI/CD and CircleCI are also excellent options, especially for teams already using GitLab or GitHub.
Step 2: Set Up Version Control
Ensure your code is in a version control system like Git. This is crucial for tracking changes and collaborating efficiently. Create a repository and encourage all team members to commit their code regularly.
Step 3: Configure the Build Pipeline
Define your build pipeline in your chosen CI tool. This typically involves specifying the steps needed to compile your code. For instance, in Jenkins, you might use a Jenkinsfile to define your pipeline. Here’s a simple example:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'make' // Command to compile your code
}
}
stage('Test') {
steps {
sh 'make test' // Command to run your tests
}
}
}
}
Step 4: Automate Testing
Automated tests are critical for CI. Write unit tests and integrate them into your build pipeline. Use testing frameworks appropriate for your programming language. For example, JUnit for Java, pytest for Python, or Mocha for JavaScript. Ensure tests run automatically on every commit.
Step 5: Monitor and Maintain
Once your CI pipeline is up and running, monitor it closely. Address any issues promptly. Regularly update your pipeline to accommodate new requirements and improvements.
Implementing CD: A Practical Guide
CD takes CI a step further by automating the deployment process. Here’s how you can implement CD:
Step 1: Define Deployment Pipelines
Define your deployment pipeline in your CD tool. This involves specifying the steps needed to deploy your application to various environments (e.g., staging, production). In Jenkins, you might extend your Jenkinsfile to include deployment stages:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'make'
}
}
stage('Test') {
steps {
sh 'make test'
}
}
stage('Deploy to Staging') {
steps {
sh 'deploy.sh staging' // Command to deploy to staging
}
}
stage('Deploy to Production') {
steps {
input 'Deploy to production?' // Manual approval
sh 'deploy.sh production' // Command to deploy to production
}
}
}
}
Step 2: Ensure Environment Parity
Ensure your staging environment mirrors your production environment. This reduces the risk of deployment issues. Use configuration management tools like Ansible, Puppet, or Chef to maintain consistency across environments.
Step 3: Implement Automated Rollbacks
Automate rollbacks to quickly revert to a previous stable version if something goes wrong. This is crucial for minimizing downtime and maintaining service reliability. Tools like Kubernetes and Helm can facilitate automated rollbacks.
Step 4: Monitor Deployments
Monitor your deployments closely. Use monitoring and logging tools like Prometheus, Grafana, and ELK Stack to keep track of deployment metrics and logs. This helps in quickly identifying and resolving issues.
Lessons from the Field
Implementing CI/CD is not without its challenges.
Start Small
Start with a small, manageable project. Implement CI/CD practices and refine your processes. Once successful, expand to larger projects. This incremental approach reduces risk and allows your team to build confidence.
Example
A software development company decided to implement CI/CD. They began with a single microservice. This microservice handled user authentication.
The team set up a CI pipeline that included automated unit tests. Every commit triggered a build and test process.
Initially, they encountered several issues. Some tests failed, and builds broke. However, these failures provided valuable lessons. The team adjusted their processes, improved test coverage, and refined their pipeline. After a few weeks, the CI pipeline was stable and reliable.
Encouraged by this success, the team extended CI/CD to other microservices. Each new implementation was smoother, thanks to the lessons learned from the initial project. Over time, the entire application benefited from CI/CD, leading to faster releases and higher quality software.
Foster a Culture of Automation
Automation should be a mindset. Encourage team members to automate repetitive tasks. Recognize and reward efforts to streamline processes. This cultural shift is crucial for the long-term success of CI/CD.
Example
In a mid-sized tech company, manual testing was a major bottleneck. Testers spent hours on repetitive tasks. The company decided to promote a culture of automation. They started a campaign to identify and automate repetitive tasks.
Developers and testers collaborated to create automated scripts for common tests. They used tools like Selenium for automated UI tests and JUnit for unit tests. Management recognized and rewarded team members who contributed significantly to automation.
Over time, the company saw a dramatic reduction in manual testing hours. Automated tests ran consistently and reliably. This shift allowed testers to focus on more complex, exploratory testing. The overall quality of the software improved, and releases became more frequent.
Invest in Training
Provide training and resources to your team. Ensure they understand CI/CD principles and tools. This investment in knowledge will pay off in smoother implementations and more effective use of CI/CD practices.
Example
A financial services company faced challenges with their CI/CD implementation. Many team members were unfamiliar with the tools and practices. The company decided to invest in comprehensive training.
They organized workshops and brought in CI/CD experts. They also provided access to online courses and resources. Team members learned about various CI/CD tools like Jenkins, GitLab CI/CD, and Docker.
They practiced setting up pipelines and automating tests.
The training had a significant impact. Team members felt more confident and competent. They applied their new knowledge to real projects, leading to more effective CI/CD pipelines. The company saw improvements in deployment speed and software quality.
Insight 4: Prioritize Security
Integrate security into your CI/CD pipelines. Use tools like Snyk or OWASP Dependency-Check to scan for vulnerabilities. Ensure compliance with security best practices throughout the development and deployment process.
Example
A healthcare software company needed to ensure the security of their applications. They integrated security checks into their CI/CD pipelines. They used tools like Snyk to scan for vulnerabilities in their dependencies.
Every time code was committed, the CI pipeline included a security scan. Any vulnerabilities found were reported immediately. Developers prioritized fixing these issues before merging code into the main branch.
Additionally, the company implemented security training for their developers. They learned about common vulnerabilities and best practices for writing secure code. This proactive approach significantly reduced security risks and ensured compliance with industry regulations.
Starting small, fostering a culture of automation, investing in training, and prioritizing security are key insights for successful CI/CD implementation. These examples demonstrate how addressing challenges with these strategies can lead to significant improvements in software development processes. Implementing CI/CD is a journey, but with the right approach, it can transform the way your team works, leading to more efficient, reliable, and secure software delivery.
Example
Let’s look at an example to illustrate the impact of CI/CD. A mid-sized e-commerce company faced challenges with frequent production issues and slow deployment cycles. They decided to implement CI/CD to address these problems.
The Challenge
The company had a monolithic codebase with infrequent, manual deployments. Each deployment was risky and error-prone. Production issues were common, causing customer dissatisfaction and revenue loss.
The Solution
The company started by breaking down the monolith into microservices. They implemented CI/CD pipelines for each microservice. Automated tests and builds were set up to run on every commit. Deployment pipelines automated the release process to staging and production environments.
The Outcome
The impact was immediate and significant. Deployment frequency increased from once a month to several times a day. Production issues decreased dramatically. The team could quickly identify and fix issues, leading to improved code quality. Customer satisfaction improved, and the company saw a positive impact on revenue.
Wrapping up
CI/CD is more than just a set of tools and practices. It’s a transformative approach to software development. By automating integration and deployment processes, CI/CD can significantly improve productivity, code quality, and deployment speed.
Teams that embrace CI/CD will find themselves more agile and better equipped to respond to changing market demands. Start small, foster a culture of automation, invest in training, and prioritize security. These steps will set you on the path to CI/CD success.
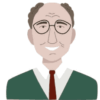
James is an esteemed technical author specializing in Operations, DevOps, and computer security. With a master’s degree in Computer Science from CalTech, he possesses a solid educational foundation that fuels his extensive knowledge and expertise. Residing in Austin, Texas, James thrives in the vibrant tech community, utilizing his cozy home office to craft informative and insightful content. His passion for travel takes him to Mexico, a favorite destination where he finds inspiration amidst captivating beauty and rich culture. Accompanying James on his adventures is his faithful companion, Guber, who brings joy and a welcome break from the writing process on long walks.
With a keen eye for detail and a commitment to staying at the forefront of industry trends, James continually expands his knowledge in Operations, DevOps, and security. Through his comprehensive technical publications, he empowers professionals with practical guidance and strategies, equipping them to navigate the complex world of software development and security. James’s academic background, passion for travel, and loyal companionship make him a trusted authority, inspiring confidence in the ever-evolving realm of technology.