DevOps and Cloud-Native strategies are at the forefront of digital transformation, offering unparalleled agility and robustness in today’s dynamic market.
In today’s fast-paced business world, companies are increasingly relying on cloud infrastructure to achieve greater agility, scalability, and cost-effectiveness.
At the same time, they are adopting DevOps methodologies to accelerate software delivery and improve collaboration between development and operations teams.
Combining the two approaches can unleash powerful synergies that transform the way organizations build, deploy, and run their applications. However, implementing DevOps in a cloud environment comes with its own set of challenges, such as ensuring security, managing configurations, and optimizing performance.
The Foundations of DevOps
DevOps is a software development approach that emphasizes collaboration, integration, and automation between software development and IT operations teams. At its core, DevOps is based on a set of principles that aim to improve the speed and quality of software delivery while reducing the risk of failures and downtime.
DevOps Principles
One of the key principles of DevOps is continuous integration (CI), which involves merging code changes regularly and automatically verifying that the resulting code can be built and tested successfully. Continuous delivery (CD) is another important principle that involves automating the deployment of software to production environments, ensuring that changes can be released quickly and reliably. Infrastructure as code (IaC) is a third principle that involves treating infrastructure configuration as code, enabling it to be versioned, tested, and deployed automatically alongside application code.
By following these principles, DevOps teams can collaborate more effectively, iterate more quickly, and respond to changing business needs with greater agility.
Continuous Integration
Continuous integration is a process that involves regularly merging code changes into a shared repository and running automated tests to ensure that the changes do not introduce errors or conflicts with existing code. CI helps to catch issues early and minimize the time and effort required to resolve them. In addition, it promotes a culture of collaboration, as developers are encouraged to work together and share code changes frequently.
Here’s an example of a basic CI pipeline using GitHub Actions. This pipeline is defined in a YAML file that is placed in a repository on GitHub. It will trigger an automated build and run tests every time a new commit is pushed to the repository.
- Create a
.github/workflows
folder in your repository. - Add a CI workflow file (e.g.,
ci.yml
) in the.github/workflows
directory. - Define the CI workflow. Below is an example
ci.yml
file for a Node.js project:
name: Node.js CI
on:
push:
branches: [ master ]
pull_request:
branches: [ master ]
jobs:
build:
runs-on: ubuntu-latest
strategy:
matrix:
node-version: [12.x, 14.x, 16.x]
steps:
- uses: actions/checkout@v2
- name: Use Node.js ${{ matrix.node-version }}
uses: actions/setup-node@v1
with:
node-version: ${{ matrix.node-version }}
- run: npm ci
- run: npm run build --if-present
- run: npm test
In this example:
name
: Specifies the name of the CI process.on
: Defines the events that trigger the workflow. In this case, it triggers on push and pull request events to the master branch.jobs
: Defines the jobs to run. Here, we have a single job namedbuild
.runs-on
: Specifies the type of machine to run the job on. Here, it uses the latest Ubuntu version.strategy
: Defines a matrix strategy to test against multiple versions of Node.js.steps
: A sequence of tasks that will be executed as part of the job. In this example, it checks out the code, sets up Node.js, installs dependencies, builds the project, and runs tests.
This is a basic example and CI configurations can become quite complex depending on the requirements of the project. CI tools support a wide range of languages and frameworks, so you would need to tailor your CI configuration to your project’s specific needs.
Continuous Delivery
Continuous delivery is a practice that involves automating the entire software release process, from building and testing to deployment and monitoring. CD enables teams to release new features quickly and reliably, reducing the risk of bugs or downtime in production environments.
By automating the deployment process, CD also frees up IT operations teams to focus on higher-value tasks, such as optimizing infrastructure and improving application performance.
Infrastructure as Code
Infrastructure as code is a practice that involves treating infrastructure configuration as code, enabling it to be versioned, tested, and deployed automatically alongside application code. IaC helps to ensure that infrastructure is consistent across different environments and reduces the risk of configuration errors or human mistakes.
It also enables teams to manage infrastructure in a more agile and efficient way, making it easier to scale resources up or down as needed.
By understanding these foundational principles of DevOps, teams can begin to implement more efficient and effective software development practices, leading to faster release cycles, better collaboration, and improved software quality.
Cloud Native Technologies
In order to fully harness the power of DevOps in a cloud environment, it is essential to embrace cloud-native technologies. These cutting-edge tools and practices are specifically designed to enable greater scalability, portability, and flexibility in the cloud, while also facilitating more efficient and collaborative development workflows.
Containers
Containers are a fundamental building block of cloud-native architecture. They provide a lightweight, portable way to package applications, along with all their dependencies, into a self-contained unit that can be easily moved between environments.
By breaking down applications into smaller, more modular components, containers allow for greater scalability and agility, while also simplifying deployment and maintenance.
Orchestration
However, managing containerized applications at scale can quickly become complex and unwieldy. That’s where orchestration tools like Kubernetes come in. Kubernetes is a highly popular open-source platform that automates the deployment, scaling, and management of containerized applications.
It provides a powerful set of features for managing clusters of hosts and allocating resources based on demand, ensuring that applications can run reliably and efficiently no matter what.
Microservices
Another key component of cloud-native architecture is the microservices approach. In contrast to traditional monolithic applications, microservices architecture breaks down complex applications into a series of smaller, loosely coupled services that can be developed, deployed, and scaled independently.
This approach enables greater agility, as developers can make changes to specific services without affecting the entire application. It also facilitates a more modular, collaborative development process, as different teams can work on different services simultaneously.
1. Microservice Example
First, let’s create a simple Python Flask application as our microservice. This microservice will provide a simple REST API.
Python Flask Microservice (app.py
):
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/')
def home():
return jsonify({"message": "Hello from Microservice"})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
2. Dockerize the Microservice
To deploy this microservice, you need to containerize it using Docker. Create a Dockerfile
to build a Docker image.
Dockerfile:
# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy the current directory contents into the container at /usr/src/app
COPY . .
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir flask
# Make port 5000 available to the world outside this container
EXPOSE 5000
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
3. Kubernetes Deployment
After building and pushing the Docker image to a container registry (like Docker Hub), you can deploy it using Kubernetes.
Kubernetes Deployment (deployment.yaml
):
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-microservice
spec:
replicas: 2
selector:
matchLabels:
app: my-microservice
template:
metadata:
labels:
app: my-microservice
spec:
containers:
- name: my-microservice
image: <your-docker-image> # Replace with your Docker image path
ports:
- containerPort: 5000
Kubernetes Service (service.yaml
):
apiVersion: v1
kind: Service
metadata:
name: my-microservice
spec:
type: LoadBalancer
selector:
app: my-microservice
ports:
- protocol: TCP
port: 80
targetPort: 5000
This Kubernetes deployment will create two replicas of the microservice and expose them via a LoadBalancer service.
Combining it All
In a real-world DevOps environment, these steps would be automated:
- The microservice code is stored in a source control system like Git.
- Upon a new commit, a CI/CD pipeline (using tools like Jenkins, GitLab CI, or GitHub Actions) builds the Docker image and pushes it to a container registry.
- The pipeline then applies the Kubernetes configurations to deploy the updated microservice to a cluster.
This example is basic and serves to illustrate the concept. Real-world microservices would be more complex, potentially involving multiple services, databases, and other integrations, each with their own CI/CD pipelines and deployment strategies.
Overall, by embracing cloud-native technologies like containers, orchestration, and microservices, enterprises can unlock unprecedented levels of scalability, agility, and efficiency in their DevOps practices. However, it’s important to recognize that these technologies also require a significant shift in mindset and culture, as well as a commitment to ongoing learning and experimentation.
Implementing DevOps in Cloud Environments
Implementing DevOps in cloud environments requires a cohesive strategy that takes into account the unique challenges of cloud infrastructure. Automated provisioning and configuration management are key to ensuring consistency and reliability of cloud-native applications. Additionally, monitoring and observability provide insights into the health and performance of cloud-based systems.
Cloud Infrastructure
A solid foundation of cloud infrastructure is essential to implementing DevOps in a cloud environment. Cloud infrastructure should be designed to provide resilience, scalability, and security. Automated provisioning enables teams to quickly and efficiently spin up new resources, reducing manual errors and improving consistency. Configuring cloud infrastructure as code ensures that infrastructure changes can be tracked and audited, providing greater accountability.
Automated Provisioning and Configuration Management
Configuration management tools enable teams to automate the process of configuration and deployment, ensuring consistency and reproducibility. These tools also facilitate testing and monitoring, allowing teams to identify and address issues quickly. Automated provisioning and configuration management can be especially beneficial in cloud environments, where resources can be quickly scaled up or down in response to demand, and changes must be made quickly and accurately.
Monitoring and Observability
As applications become more complex and distributed, monitoring and observability become increasingly critical. In a cloud-native environment, monitoring and observability provide insights into the health and performance of cloud-based systems.
Teams can use monitoring to identify issues before they become critical, and observability to gain insight into the behavior of distributed systems. Effective monitoring and observability provide teams with the data they need to identify and resolve issues quickly, and to improve overall system performance.
CI/CD Pipelines in a Cloud Native Context
CI/CD pipelines are vital components of cloud-native DevOps practices. By automating the build, test, and deployment processes, businesses can achieve faster time-to-market and greater agility.
Version Control
Version control is an essential aspect of CI/CD pipelines. By leveraging tools such as Git, teams can ensure the traceability and reproducibility of code changes. This enables developers to collaborate more effectively and reduces the risk of errors caused by conflicting changes.
Automated Testing
Automated testing is a critical step in guaranteeing the quality and stability of cloud-native applications. By automating unit tests, integration tests, and end-to-end tests, developers can catch issues early and ensure that applications meet the expected requirements. This also helps to reduce the chances of bugs making their way into production.
Deployment Strategies
There are different deployment strategies available to enterprises when implementing CI/CD pipelines. For instance, in the blue-green deployment method, two identical environments are created, where one is active and the other is idle. The latest version of an application is deployed to the idle environment and tested thoroughly before routing traffic to it.
This ensures minimal downtime and faster rollbacks if necessary. Canary release is another method where a small subset of users are directed to the new release to test its features and performance before a full release.
Scalability and Resilience in Cloud-Native DevOps
In a cloud-native DevOps environment, scalability and resilience are critical components for ensuring the successful deployment and operation of applications. Scalability refers to the ability of an application to handle an increasing amount of workload, while resilience pertains to the ability to withstand and recover quickly from failures.
Auto-scaling is a core feature of cloud-native infrastructure that allows resources to be automatically provisioned as demand increases, and de-provisioned when it decreases. This ensures that the application can handle varying workloads efficiently, providing a seamless user experience. By leveraging auto-scaling mechanisms, enterprises can also save costs by only paying for the resources they need.
Fault tolerance is another key aspect of resilience, which involves designing the system to handle failures gracefully. This can be achieved by ensuring that critical components of the application have redundancy and failover mechanisms in place. In the event of a failure, these systems can detect the issue and automatically initiate the failover process, preventing downtime and data loss.
Designing for Scalability
To design an application for scalability, it is essential to consider the following factors:
- The ability to horizontally scale out by adding more instances of the application
- The use of caching mechanisms to reduce the amount of requests to back-end services
- The use of stateless architecture, to ensure that requests can be handled by any instance of the application
Ensuring Resiliency
When designing for resiliency, it is important to:
- Ensure that all data is stored in a highly available and durable storage solution to prevent data loss
- Implement back-up and restore mechanisms, to provide disaster recovery in case of failures
- Ensure that the application can handle increased traffic by leveraging the auto-scaling capabilities of the cloud-native infrastructure
Scalability and resilience are essential components of a cloud-native DevOps environment. By leveraging auto-scaling and fault tolerance mechanisms, enterprises can ensure that their applications are always up and running, providing a seamless experience to their users.
Security Considerations in Cloud-Native DevOps
As cloud-native DevOps continues to gain momentum, it’s essential to consider the security implications of deploying applications in a cloud environment. Here are some security best practices to keep in mind:
Identity and Access Management
Implementing strong identity and access management (IAM) controls is crucial in ensuring that only authorized users can access your cloud resources. Use role-based access control (RBAC) to grant permissions based on job functions, and enforce strong password policies to prevent unauthorized access.
Network Security
Securing network communications between different cloud resources and services is vital in cloud-native DevOps. Use virtual private networks (VPNs) to establish secure connections between your cloud services and on-premises infrastructure. Implement firewalls to control inbound and outbound traffic and enable logging to track any unusual activity.
Compliance
Adhering to industry regulations and standards is critical in a cloud-native DevOps setting. Ensure that your cloud infrastructure and services meet compliance requirements for data privacy, security, and governance. Regularly conduct compliance audits and assessments to identify and address any potential vulnerabilities.
Continuous Monitoring
Continuous monitoring of your cloud environment is an essential component of cloud-native DevOps. Use automated tools to track any suspicious activity or unauthorized access attempts. Set up alerts to notify you of any security breaches and conduct regular vulnerability scans to identify and address any weaknesses in your cloud infrastructure.
By following these security best practices, you can ensure the safety and security of your cloud-native DevOps environment and protect your organization from potential security threats.
Tools and Technologies for Cloud-Native DevOps
Cloud-native DevOps practices rely heavily on automation and collaboration. Fortunately, there are numerous DevOps tools available that can assist in streamlining and optimizing processes. Let’s explore some popular DevOps tools and cloud-native tooling that can help organizations achieve their cloud-native DevOps goals.
DevOps Tools
Some of the most widely used DevOps tools include:
Tool | Description |
---|---|
Git | A version control system that allows for efficient collaboration and management of code changes. |
Jenkins | An open-source automation server that enables continuous integration and continuous delivery of software. |
Ansible | A configuration management tool that automates software deployment, configuration, and management. |
Cloud-Native Tooling
Organizations can leverage a variety of cloud-native tooling to manage applications in cloud environments. These include:
- Container registries: Tools like Docker Hub and Amazon ECR provide secure storage and distribution of container images.
- Orchestration tools: Kubernetes is a popular open-source tool for managing containerized applications and automating deployment, scaling, and management.
- Continuous monitoring tools: Tools like New Relic and Prometheus enable real-time monitoring of cloud-native applications and infrastructure.
Continuous Monitoring and Log Management
Continuous monitoring and effective log management are essential components of a cloud-native DevOps environment. By leveraging tools like New Relic or LogDNA, organizations can gain insight into application performance and quickly troubleshoot issues. Log management tools can assist in consolidating logs from different sources and making them easily searchable and understandable.
With the right tools and technologies, organizations can successfully implement cloud-native DevOps practices and reap the benefits of enhanced agility, scalability and efficiency.
Harnessing the Power of DevOps in Cloud Native Environments
The benefits of combining DevOps and cloud-native practices are becoming increasingly evident as organizations seek to enhance their agility, scalability, and efficiency. Adopting DevOps in a cloud-native environment allows enterprises to streamline their development and operations processes, facilitate automation and collaboration, and leverage cutting-edge technologies for greater innovation.
Moreover, the future looks bright for cloud-native DevOps, as more and more businesses are recognizing the potential of this approach and investing in it accordingly. Emerging trends, such as serverless computing and edge computing, offer exciting possibilities for further optimizing the performance and resilience of cloud-native applications.
Overall, the key takeaway is that DevOps adoption in a cloud-native environment is a winning strategy for any organization that aims to stay ahead of the curve in today’s digital landscape. By embracing the powerful combination of DevOps principles and cloud-native technologies, enterprises can unlock new levels of efficiency, productivity, and innovation.
External Resources
FAQ
1. What is the role of containers in Cloud-Native DevOps?
Answer: Containers play a crucial role in Cloud-Native DevOps by providing a lightweight, consistent, and isolated environment for applications. They allow for easy packaging and deployment of applications across different environments, ensuring consistency and reliability.
Code Sample: Creating a Dockerfile for a simple Node.js application:
# Use an official Node runtime as a parent image
FROM node:14
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install any needed packages specified in package.json
RUN npm install
# Bundle app source inside the container
COPY . .
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.js when the container launches
CMD ["node", "app.js"]
2. How does Infrastructure as Code (IaC) support Cloud-Native DevOps?
Answer: IaC is a key practice in Cloud-Native DevOps that involves managing and provisioning infrastructure through machine-readable definition files, rather than physical hardware configuration or interactive configuration tools. It increases efficiency, consistency, and scalability in cloud environments.
Code Sample: Example of a Terraform script to create an AWS EC2 instance:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "ExampleInstance"
}
}
3. What is a CI/CD pipeline, and why is it important in Cloud-Native DevOps?
Answer: CI/CD stands for Continuous Integration/Continuous Deployment. In Cloud-Native DevOps, a CI/CD pipeline automates the process of integrating code changes from multiple developers, testing them, and then deploying them to production environments. This ensures faster and more reliable deliveries.
Code Sample: GitLab CI/CD pipeline configuration (.gitlab-ci.yml
):
stages:
- build
- test
- deploy
build_job:
stage: build
script:
- echo "Building the application..."
- build_script
test_job:
stage: test
script:
- echo "Running tests..."
- test_script
deploy_job:
stage: deploy
script:
- echo "Deploying to production..."
- deploy_script
4. How do microservices architecture and DevOps complement each other in Cloud-Native applications?
Answer: Microservices architecture aligns well with DevOps practices in Cloud-Native applications by enabling small, independent teams to develop, deploy, and scale their services independently. This leads to faster development cycles, easier maintenance, and better scalability.
Code Sample: Docker Compose file to set up a simple microservices architecture:
version: '3'
services:
web:
image: my-web-app
ports:
- "5000:5000"
api:
image: my-api
ports:
- "4000:4000"
5. What are some best practices for monitoring and logging in Cloud-Native DevOps?
Answer: Best practices include implementing centralized logging, real-time monitoring, alerting, and integrating these tools into the CI/CD pipeline. This approach ensures visibility, helps in quick debugging, and maintains the health of Cloud-Native applications.
Code Sample: Configuration snippet for a Prometheus monitoring setup:
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'prometheus'
static_configs:
- targets: ['localhost:9090']
These questions cover fundamental aspects of DevOps and Cloud-Native practices, providing a good starting point for understanding and implementing these concepts in practical scenarios.
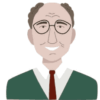
James is an esteemed technical author specializing in Operations, DevOps, and computer security. With a master’s degree in Computer Science from CalTech, he possesses a solid educational foundation that fuels his extensive knowledge and expertise. Residing in Austin, Texas, James thrives in the vibrant tech community, utilizing his cozy home office to craft informative and insightful content. His passion for travel takes him to Mexico, a favorite destination where he finds inspiration amidst captivating beauty and rich culture. Accompanying James on his adventures is his faithful companion, Guber, who brings joy and a welcome break from the writing process on long walks.
With a keen eye for detail and a commitment to staying at the forefront of industry trends, James continually expands his knowledge in Operations, DevOps, and security. Through his comprehensive technical publications, he empowers professionals with practical guidance and strategies, equipping them to navigate the complex world of software development and security. James’s academic background, passion for travel, and loyal companionship make him a trusted authority, inspiring confidence in the ever-evolving realm of technology.