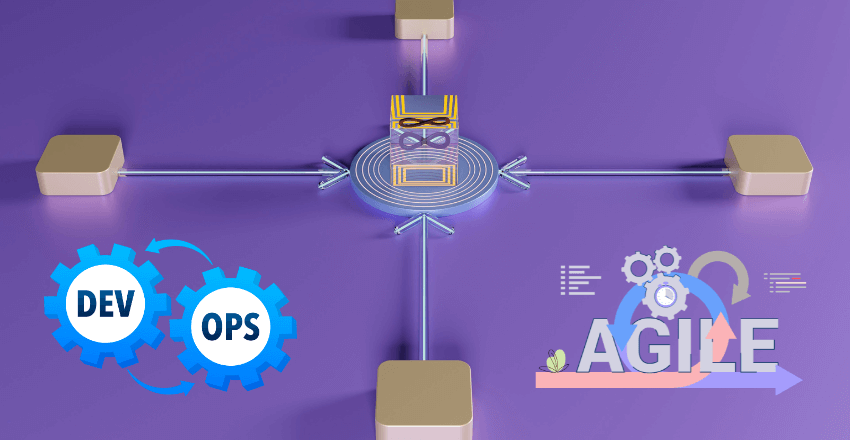
Merging Agile and DevOps transforms traditional software development, introducing a seamless pipeline from concept to deployment with enhanced teamwork.
DevOps and Agile methodologies have proven to be highly effective in their respective domains. However, organizations are increasingly recognizing the benefits of merging Agile and DevOps practices to create a more efficient and collaborative software development environment.
DevOps and Agile Methodologies

While DevOps and Agile are often used interchangeably, they are actually two distinct methodologies with their own unique characteristics and goals. Understanding these methodologies is crucial to successfully integrating them into an Agile DevOps workflow.
DevOps is a software development methodology that promotes collaboration and communication between development and operations teams. Its primary goal is to streamline and automate the software development process, allowing teams to deliver high-quality software faster and more efficiently.
DevOps emphasizes continuous integration and continuous delivery (CI/CD) and encourages teams to work together to identify and resolve issues as quickly as possible.
Agile, on the other hand, is an iterative and flexible approach to software development. It emphasizes customer satisfaction and the ability to respond quickly to changing requirements and priorities. Agile teams work in short sprints, delivering working software at the end of each iteration.
They prioritize collaboration, feedback, and continuous improvement, and value individuals and interactions over processes and tools.
Integrating DevOps and Agile methodologies into a cohesive workflow can significantly enhance the software development process, making it more efficient, responsive, and user-centered.
Below is a simplified code example that demonstrates how you might automate the build, test, and deployment processes within an Agile sprint using GitHub Actions, a CI/CD tool that fits into the DevOps philosophy.
This example assumes you have a simple web application written in Node.js and you want to automate its testing and deployment to a cloud service (e.g., Heroku) whenever changes are pushed to the main branch, ensuring continuous integration and delivery are in place within your Agile framework.
GitHub Actions Workflow for CI/CD
Create a .github/workflows/ci-cd.yaml
file in your repository:
name: Node.js CI/CD Pipeline
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
strategy:
matrix:
node-version: [14.x]
steps:
- uses: actions/checkout@v2
- name: Use Node.js ${{ matrix.node-version }}
uses: actions/setup-node@v1
with:
node-version: ${{ matrix.node-version }}
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
- name: Build
run: npm run build
deploy:
needs: build
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Deploy to Heroku
uses: akhileshns/heroku-deploy@v3.12.12 # This action simplifies deployment to Heroku
with:
heroku_api_key: ${{ secrets.HEROKU_API_KEY }}
heroku_app_name: "your-app-name" # Replace with your Heroku app name
heroku_email: "your-email@example.com" # Replace with your Heroku account email
usedocker: true
Explanation:
- On Push Trigger: The workflow is triggered whenever changes are pushed to the
main
branch, aligning with Agile’s iterative nature by ensuring new features and fixes are continuously integrated and delivered. - Build Job: It sets up the Node.js environment, installs dependencies, runs tests, and builds the application. This step ensures that every change is tested and buildable, maintaining code quality and stability.
- Deploy Job: If the build job succeeds, the deployment job begins. It uses a GitHub Action to deploy the application to Heroku, automating the deployment process. This step embodies DevOps by streamlining deployment, reducing manual intervention, and enabling faster delivery to the end-users.
This CI/CD pipeline is a practical example of merging Agile and DevOps. Agile’s focus on iterative development, customer feedback, and adaptability, combined with DevOps’ emphasis on automation, collaboration, and quick deliveries, ensures a robust, efficient, and user-centric development lifecycle.
When combined, DevOps and Agile can create a powerful and efficient software development workflow.
DevOps can provide the automation and efficiency necessary to deliver software quickly and reliably, while Agile can ensure that the software being developed meets the needs of the end-users and is continually improved based on their feedback.
The Role of Collaboration in Agile DevOps
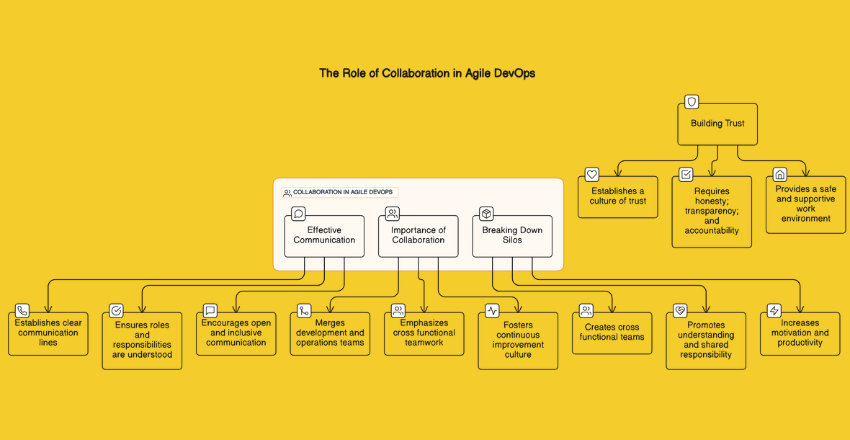
Collaboration is crucial for achieving success in Agile DevOps. As DevOps emphasizes the collaboration between development and operations teams, merging Agile methodologies into the mix further emphasizes the need for cross-functional teams that work together seamlessly. Effective communication and collaboration can foster a culture of continuous improvement, leading to high-quality, delivered products.
Breaking Down Silos
The first step to achieving collaborative success in Agile DevOps is to break down silos between development, operations, and other teams. Silos lead to a lack of communication, preventing cross-functional teams from working together and sharing knowledge. This can cause missed opportunities to streamline processes and identify areas for improvement.
Breaking down silos involves creating cross-functional teams that work collaboratively towards a shared goal. A cross-functional approach can promote an understanding of each team’s perspectives, leading to more effective communication and collaboration. Cross-functional teams can also encourage a sense of ownership and shared responsibility, leading to increased motivation and productivity.
Effective Communication
Effective communication is fundamental for Agile DevOps. This means establishing clear lines of communication and ensuring that everyone understands their roles and responsibilities. Establishing open communication channels can help to identify issues early and prevent misunderstandings that can lead to delays.
It is also important to ensure that communication channels are inclusive and accessible, so that all team members can provide input and feedback. Encouraging open communication can also lead to the creation of a culture of trust, which is essential for creating a collaborative and supportive work environment.
Building Trust
Building trust is a fundamental component of successful collaboration in Agile DevOps. This means establishing a culture of trust, where team members can rely on one another and collectively work towards a shared goal. Building trust requires honesty, transparency, and accountability. It is important to ensure that team members feel safe to share their ideas, feedback, and opinions.
Encouraging trust also means providing a safe and supportive work environment. This can involve providing team members with the resources and tools they need to succeed. It may also involve providing training and development opportunities to help team members improve their skills and abilities.
Overall, collaboration is essential for Agile DevOps success. By breaking down silos, establishing effective communication, and building trust, organizations can create cross-functional, collaborative teams that work seamlessly towards a shared goal.

Integrating Agile Practices into DevOps
Agile practices can be seamlessly integrated into the DevOps workflow to improve efficiency and effectiveness. By leveraging Agile principles such as continuous integration and frequent iterations, DevOps teams can achieve faster delivery and higher quality results.
Continuous Integration (CI) is a foundational Agile practice that ensures code is tested and integrated into a shared repository multiple times a day. This helps identify any issues early on in the development process, reducing the cost and time spent debugging later on.
CI is also a critical component of DevOps, as it allows teams to deliver new features quickly and efficiently.
Frequent iterations are another key Agile practice that can be incorporated into DevOps. By breaking down work into smaller, manageable pieces, teams can iterate on features, receive feedback, and adjust their approach as needed. This helps ensure that the final product meets the needs of stakeholders and is delivered on time.
Other Agile practices that can be integrated into DevOps include:
- Automated testing
- Self-organizing teams
- Customer collaboration
- Timeboxing
Integrating Agile practices into a DevOps environment enhances the collaboration, speed, and quality of software development and deployment.
Below is a practical code example demonstrating how to incorporate Continuous Integration (CI) and frequent iterations into a DevOps pipeline using Jenkins, a popular automation server that supports Agile principles.
This example outlines a Jenkinsfile for a Java-based project, which automates the process of building, testing, and deploying an application. This Jenkins pipeline is designed to be triggered on every commit, embodying the Agile practices of CI and frequent iterations.
Jenkinsfile for Agile Practices Integration
pipeline {
agent any
environment {
// Define environment variables for consistency across stages
JAVA_HOME = "/usr/lib/jvm/java-11-openjdk"
}
stages {
stage('Checkout') {
steps {
// Checkout source code from version control system
checkout scm
}
}
stage('Build') {
steps {
// Compile the project
sh './mvnw clean package'
}
}
stage('Test') {
steps {
// Run automated tests
sh './mvnw test'
}
}
stage('Deploy') {
steps {
// Deploy the application to a staging environment for further validation
sh 'echo Deploying to staging environment'
// Example command for deployment
// sh './deploy-to-staging.sh'
}
}
}
post {
always {
// Clean up workspace after the pipeline runs to maintain a clean environment
cleanWs()
}
success {
// Actions to take on successful pipeline completion
echo 'Build, Test and Deployment stages have completed successfully.'
}
failure {
// Actions to take if the pipeline fails at any stage
echo 'An error has occurred during one of the stages.'
}
}
}
Explanation:
- Environment Setup: Defines the Java home environment variable to ensure consistency in builds across different stages of the pipeline.
- Checkout Stage: Retrieves the latest code from the source control management (SCM) system, which is crucial for CI as it ensures the most recent changes are integrated and tested.
- Build Stage: Compiles the project using Maven, a build automation tool. This stage represents an Agile iteration, where new features or changes are prepared for testing.
- Test Stage: Executes automated tests using Maven. This is a core practice of Agile, emphasizing the importance of testing early and often to catch issues as soon as possible.
- Deploy Stage: Deploys the application to a staging environment. This step is vital for receiving early feedback on the deployment aspect of the application, aligning with Agile’s focus on customer collaboration and feedback.
- Post Actions: Include cleanup, success, and failure handling to maintain a clean environment and provide feedback on the pipeline’s status, promoting continuous improvement.
By following this Jenkins pipeline example, teams can effectively integrate Agile practices into their DevOps workflow, achieving faster delivery times, higher quality products, and a more responsive development process.
By incorporating these Agile practices into their DevOps workflow, organizations can foster a culture of continuous improvement and achieve greater success in their software development initiatives.
Automation in Agile DevOps
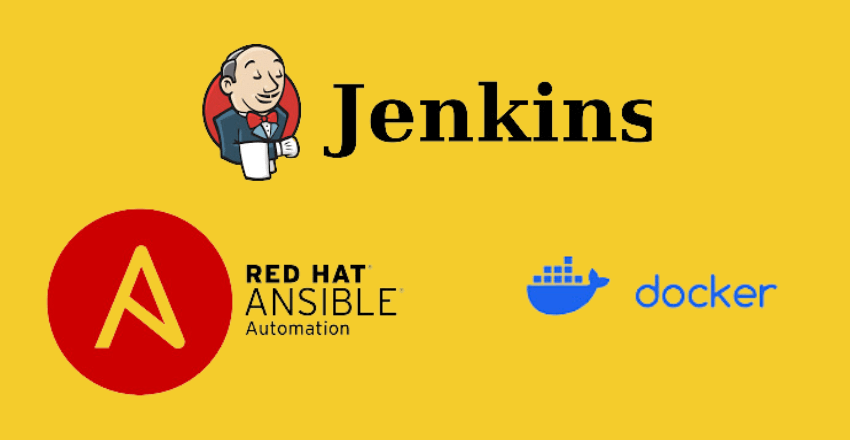
Automation plays a crucial role in Agile DevOps, helping to streamline processes, reduce errors, and improve efficiency. By automating repetitive and time-consuming tasks, teams can focus on higher-value activities such as innovation and building new features.
Some of the popular automation tools used in an Agile DevOps environment include:
Tool Name | Description |
---|---|
Jenkins | An open-source automation server used for continuous integration and continuous delivery. |
Ansible | An open-source automation tool used for software provisioning, configuration management, and application deployment. |
Docker | An open-source containerization platform that helps to automate the deployment of applications in a DevOps environment. |
However, automation is not a silver bullet. It is important to avoid over-automation and strike a balance between manual and automated testing. Additionally, automation should not replace human judgment and decision-making in critical areas such as security and compliance.
Leveraging automation within Agile DevOps practices is essential for enhancing the speed and quality of software development and delivery. This code sample demonstrates a simple, yet comprehensive, automation workflow combining Jenkins for Continuous Integration/Continuous Delivery (CI/CD), Ansible for configuration management, and Docker for containerization.
The workflow illustrates how to automate the process of building, testing, deploying a simple Python web application, and managing its configuration, encapsulating core Agile DevOps automation principles.
Jenkinsfile (Jenkins Pipeline)
This Jenkinsfile sets up a CI/CD pipeline for a Python web application. It includes stages for building the Docker image, running tests, and deploying the application.
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
// Building Docker Image
sh 'docker build -t python-app .'
}
}
}
stage('Test') {
steps {
script {
// Running tests inside the Docker container
sh 'docker run --rm python-app pytest'
}
}
}
stage('Deploy') {
steps {
script {
// Deploy using Ansible playbook
sh 'ansible-playbook -i inventory deploy_app.yml'
}
}
}
}
}
Dockerfile (Containerization)
This Dockerfile specifies the environment for a Python web application, demonstrating how Docker can be used to containerize applications in a DevOps workflow.
# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy the current directory contents into the container at /usr/src/app
COPY . .
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
Ansible Playbook (Configuration Management and Deployment)
This Ansible playbook demonstrates how to deploy the Docker container to a server, showcasing Ansible’s role in automating deployment and configuration management.
---
- name: Deploy Python web application
hosts: all
become: yes
tasks:
- name: Pull the latest version of the Docker image
docker_image:
name: "python-app"
source: pull
- name: Stop the previous container
docker_container:
name: "python-app"
state: absent
- name: Start the new container
docker_container:
name: "python-app"
image: "python-app"
state: started
ports:
- "80:80"
Notes on Balance Between Automation and Manual Efforts
While this example showcases how to automate the build, test, and deployment phases of a Python web application, it’s crucial to remember the balance between automation and manual oversight.
Automation accelerates development and deployment processes, but manual review and decision-making remain essential for addressing nuanced issues like security vulnerabilities, complex configurations, and critical business logic.
Effective Agile DevOps practices involve using tools like Jenkins, Ansible, and Docker to automate where it adds value, while also ensuring that teams are involved in key decision points to maintain quality, security, and compliance.
“Automation is the key to unlock the door to the future of Agile DevOps. But it is important to use it judiciously and in moderation for best results.”
Overcoming Challenges in Harmonizing DevOps and Agile
As with any organizational change, merging Agile and DevOps can present challenges. While DevOps focuses on continuous delivery and high-speed cycles, Agile emphasizes flexibility and iteration. Some organizations may struggle to integrate the two seamlessly.
One of the biggest challenges is aligning the different priorities and goals of Agile and DevOps teams. Development teams may focus on delivering quality software while operations teams are concerned with maintaining system stability. These different objectives can lead to conflicts and delays.
Another challenge is adapting to the fast and continuous pace of Agile DevOps. Many organizations are accustomed to a slower, more traditional development process. Incorporating Agile DevOps requires a cultural shift and a willingness to embrace change.
To overcome these challenges, organizations should prioritize communication and collaboration between teams. Encourage teams to work together throughout the entire development process, from planning and design to delivery and feedback. Establish clear lines of communication and encourage cross-functional teams to work together on projects.
It’s also essential to establish clear goals and performance metrics that align with both Agile and DevOps methodologies. These metrics should be tracked and regularly reviewed to ensure that teams are progressing towards their goals and delivering high-quality software.
Ultimately, the key to successful harmonization of DevOps and Agile is to create a culture of continuous improvement. Encourage teams to experiment, learn, and grow. Use feedback and data to identify areas for improvement and adapt processes accordingly. With a commitment to collaboration, flexibility, and continuous improvement, organizations can overcome the challenges of merging Agile and DevOps and reap the benefits of streamlined, high-quality software development.

Continuous Integration and Continuous Delivery in Agile DevOps
Continuous integration (CI) and continuous delivery (CD) are critical components of Agile DevOps. CI is the practice of regularly merging code changes into a central repository and conducting automated tests to detect and resolve conflicts. CD is the practice of automating the entire software release process, from building and testing to deployment and delivery.
Implementing CI/CD in an Agile DevOps environment can significantly improve software quality, reduce costs, and accelerate time-to-market. By regularly integrating and testing code changes, teams can catch and fix bugs and conflicts early on, preventing them from turning into larger, more costly issues down the line. CD streamlines the release process, eliminating manual errors and reducing deployment time.
Benefits of CI/CD in Agile DevOps
At its core, CI/CD is all about speed, efficiency, and quality. By adopting these practices, teams can enjoy a number of benefits, including:
- Faster time-to-market: CI/CD streamlines the software development process, allowing teams to release new features and updates more quickly.
- Higher quality releases: By catching and fixing bugs and conflicts early on, teams can ensure that their releases are stable and reliable.
- Reduced costs: Automating the release process can significantly reduce the time and resources needed to deploy code changes.
- Improved collaboration: CI/CD encourages cross-functional teams to work together, promoting effective communication and collaboration.
Challenges of CI/CD in Agile DevOps
While the benefits of CI/CD are clear, implementing these practices in an Agile DevOps environment does come with some challenges. For example:
- Complexity: Setting up the necessary infrastructure and tools for CI/CD can be complex and time-consuming.
- Resistance to change: Traditional development and release processes may be deeply ingrained in an organization’s culture, making it difficult to adopt new practices.
- Dependencies: In a complex software architecture, it can be challenging to manage dependencies and ensure that different components are integrated and tested correctly.
Best Practices for CI/CD in Agile DevOps
To successfully implement CI/CD in an Agile DevOps environment, teams should follow several best practices:
- Automate everything: From building and testing to deployment and delivery, automate as many processes as possible to reduce errors and save time.
- Implement version control: Use a reliable version control system to manage code changes and ensure that teams are working on the same version of the codebase.
- Ensure code quality: Conduct regular code reviews and use automated testing tools to ensure that code changes meet the organization’s quality standards.
- Encourage collaboration: Foster a culture of collaboration and communication, encouraging teams to work together to solve problems and share information.
By following these best practices, teams can overcome the challenges of CI/CD and reap the benefits of these critical Agile DevOps practices.
Measurement and Feedback in Agile DevOps
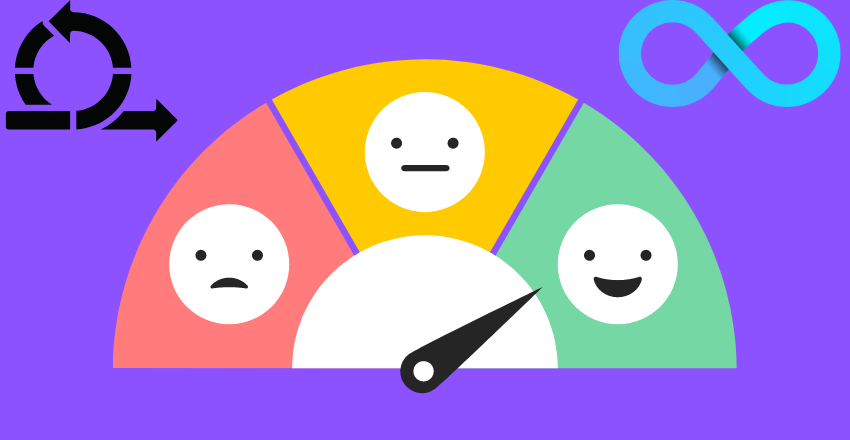
Tracking key metrics and obtaining feedback from stakeholders is essential in Agile DevOps. It helps identify areas for improvement, drive continuous growth, and deliver excellence.
One critical metric to track is the lead time for changes. It measures the time it takes to go from an idea to an implemented change. A shorter lead time is preferable, as it indicates faster delivery and faster feedback cycles. Other key metrics to track include:
- Deployment frequency: How often code changes are pushed to production
- Change fail percentage: The rate of changes that cause issues in production
- Mean time to recover: The duration between detecting and resolving production incidents
It’s crucial to obtain feedback from stakeholders throughout the software development lifecycle. Feedback can come from customer feedback, product owners, or internal stakeholders. Regular feedback cycles improve communication, ensure alignment with customer expectations, and guide decision-making.
It’s also essential to establish a culture that supports feedback. Teams should be receptive to feedback, encourage it, and act upon it. Continuous feedback loops and a willingness to iterate and improve lead to better products and more satisfied customers.
Security and Quality Assurance in Agile DevOps
Security and quality assurance are critical components of Agile DevOps. As organizations adopt DevOps practices, it is essential to ensure that security and quality are integrated throughout the software development lifecycle. This section focuses on best practices for merging Agile and DevOps with security and quality assurance at the forefront.
Security: Security is an ongoing concern for any software development project. In Agile DevOps environments, security must be integrated into the development process from the start. DevOps teams can use automation tools to identify and mitigate security vulnerabilities early in the development cycle. Additionally, incorporating security testing into the testing phase can help ensure that the final product is secure.
Quality Assurance: Quality assurance is also critical in Agile DevOps environments. Automation tools can be utilized to ensure consistency in testing, and developers should be responsible for testing their code as soon as it is written. Continuous integration and continuous delivery facilitate greater transparency throughout the development and testing process, which enables teams to identify and fix issues in real-time.
Ultimately, Agile DevOps teams must prioritize security and quality assurance to deliver secure, high-quality applications. By integrating these practices into the development process, organizations can ensure that the final product meets or exceeds customer expectations.
Final Thoughts

Harmonizing DevOps and Agile methodologies can greatly benefit organizations by improving collaboration, efficiency, and delivery excellence. By understanding the individual characteristics and goals of each methodology, organizations can successfully integrate and reap the benefits of Agile DevOps.
While challenges may arise when merging DevOps and Agile, such as potential conflicts in priorities and practices, these can be overcome through effective communication, collaboration, and by incorporating Agile practices into the DevOps workflow.
Automation, continuous integration and delivery, measurement and feedback, security, and quality assurance are all important components of Agile DevOps. By utilizing these practices, organizations can streamline processes, reduce errors, and ensure the delivery of high-quality and secure software.
OPSPros’ English-Speaking DevOps Developers
If your organization is looking to implement Agile DevOps, consider OPSPros’ dedicated English-speaking DevOps Developers. With expertise in both DevOps and Agile methodologies, they can assist your organization in successfully harmonizing these practices and achieving continuous growth and delivery excellence. Contact OPSPros today to learn more.
External Resources
FAQ
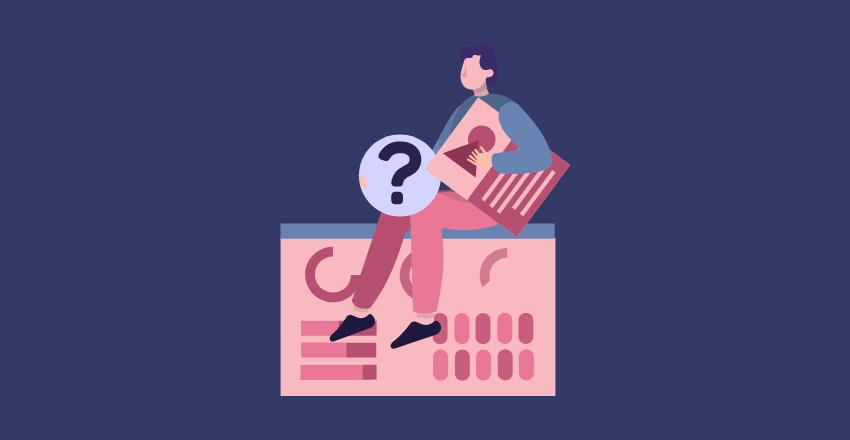
1. How can we integrate continuous integration (CI) into Agile sprints?
FAQ: How can continuous integration be integrated within Agile sprints to enhance development efficiency?
Answer: Integrating CI into Agile sprints involves automating the build and testing process every time a team member commits changes to the version control repository. This ensures that code is always in a deployable state, facilitating rapid iterations. Here’s a simple example using Jenkins, a popular CI tool:
pipeline {
agent any
stages {
stage('Build') {
steps {
// Use your build tool e.g., Maven, Gradle
sh 'mvn clean package'
}
}
stage('Test') {
steps {
// Run unit tests
sh 'mvn test'
}
}
stage('Deploy') {
steps {
// Deploy to a staging environment
sh 'echo Deploying to staging server'
}
}
}
}
This Jenkins pipeline script automates the build, test, and deploy stages, aligning with Agile sprints by providing immediate feedback on the impact of changes.
2. How do you manage configuration as code in a DevOps-enabled Agile environment?
FAQ: What strategies are recommended for managing configuration as code in a DevOps-enabled Agile environment?
Answer: Managing configuration as code involves storing configuration files in a version control system, allowing you to automate the setup and deployment of environments. This practice is crucial for consistency and traceability across different stages of the development lifecycle. Here’s an example using Terraform for infrastructure as code:
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "ExampleInstance"
}
}
This Terraform script defines an AWS EC2 instance configuration, ensuring that infrastructure setup can be automated and replicated precisely, facilitating a seamless Agile and DevOps workflow.
3. How can Agile and DevOps practices improve automated testing?
FAQ: In what ways do Agile and DevOps practices enhance the efficiency and effectiveness of automated testing?
Answer: Agile and DevOps encourage early and frequent testing, making automated testing a cornerstone of the development process. This ensures that defects are discovered and addressed sooner, reducing the cost and effort required for fixes. Here’s an example using Selenium for automated web testing:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://example.com")
# Test case: Verify the homepage title
assert "Example Domain" in driver.title
driver.quit()
Incorporating this automated test into the CI/CD pipeline ensures that it runs with every code commit, aligning with Agile’s iterative approach and DevOps’s automation goals.
4. How does containerization support Agile and DevOps practices?
FAQ: How is containerization pivotal in aligning Agile and DevOps practices for better software delivery?
Answer: Containerization, using tools like Docker, encapsulates an application’s environment, ensuring consistency across development, testing, and production. This supports Agile’s iterative development and DevOps’s continuous delivery by making deployments faster and more reliable. Here’s a Docker example:
FROM python:3.8-slim
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
CMD ["python", "app.py"]
This Dockerfile creates a container for a Python application, standardizing its environment and dependencies, facilitating smoother iterations and deployments.
5. How to implement monitoring and feedback loops in Agile DevOps?
FAQ: What approaches are recommended for implementing effective monitoring and feedback loops in an Agile DevOps environment?
Answer: Implementing monitoring and feedback loops involves using tools like Prometheus and Grafana to collect metrics and visualize system performance in real-time. This enables teams to quickly identify and address issues, aligning with Agile’s emphasis on adaptability and DevOps’s focus on operational excellence. Here’s a basic Prometheus configuration example:
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'example'
static_configs:
- targets: ['localhost:9090']
By integrating this monitoring setup, teams can continuously track application performance, supporting rapid iteration and improvement in line with Agile and DevOps principles.

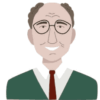
James is an esteemed technical author specializing in Operations, DevOps, and computer security. With a master’s degree in Computer Science from CalTech, he possesses a solid educational foundation that fuels his extensive knowledge and expertise. Residing in Austin, Texas, James thrives in the vibrant tech community, utilizing his cozy home office to craft informative and insightful content. His passion for travel takes him to Mexico, a favorite destination where he finds inspiration amidst captivating beauty and rich culture. Accompanying James on his adventures is his faithful companion, Guber, who brings joy and a welcome break from the writing process on long walks.
With a keen eye for detail and a commitment to staying at the forefront of industry trends, James continually expands his knowledge in Operations, DevOps, and security. Through his comprehensive technical publications, he empowers professionals with practical guidance and strategies, equipping them to navigate the complex world of software development and security. James’s academic background, passion for travel, and loyal companionship make him a trusted authority, inspiring confidence in the ever-evolving realm of technology.